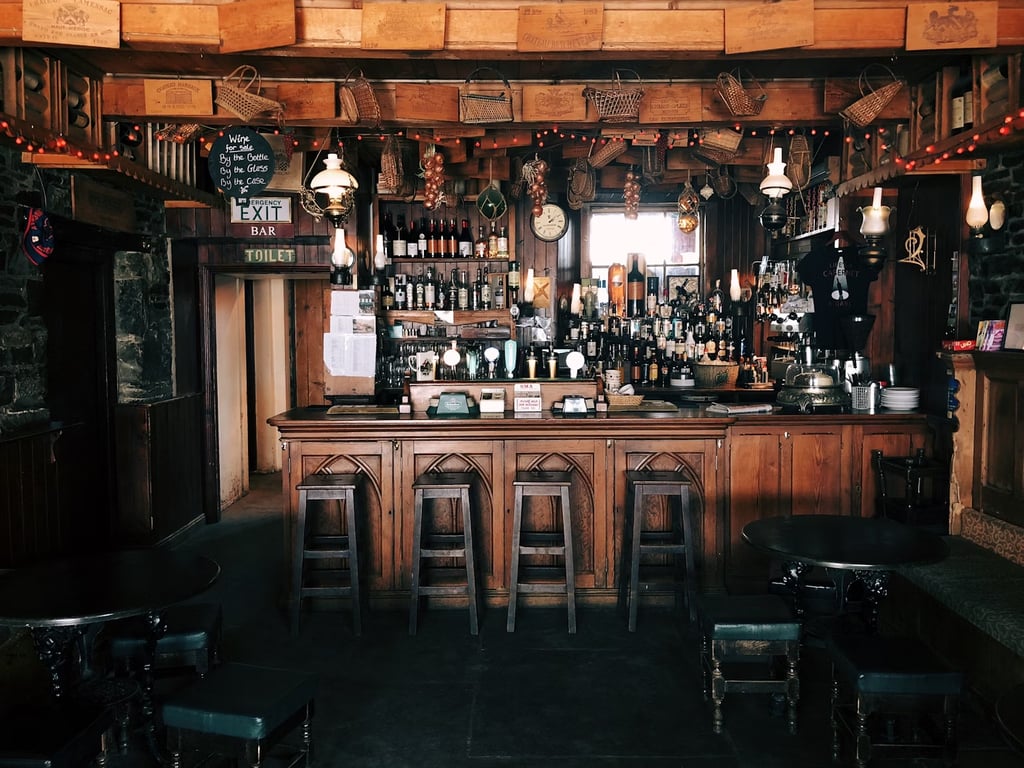
Photo by Jon Tyson on Unsplash
Python is one of the easiest programming languages to learn and master, thanks to its simplicity and versatility. Whether you’re a beginner or an experienced developer exploring Python for the first time, understanding its variables, data types, and input/output (I/O) is essential.
In this blog, we’ll explore the fundamentals of Python programming, starting with variables, diving into the various data types, and mastering input/output operations. By the end, you’ll be equipped with a solid foundation to start your Python journey.
What Are Variables in Python?
Definition
A variable in Python is a symbolic name that represents a value stored in memory. Variables are used to store data, which can then be manipulated or retrieved.
How to Declare a Variable
In Python, declaring a variable is as simple as assigning a value to it using the =
operator. There is no need to explicitly declare the type of the variable, as Python is dynamically typed.
# Declaring variables
name = "Alice" # String
age = 25 # Integer
height = 5.7 # Float
# Printing variables
print(name) # Output: Alice
print(age) # Output: 25
print(height) # Output: 5.7
Rules for Naming Variables
Variable names must start with a letter or an underscore (_).
They can only contain letters, numbers, and underscores.
Variable names are case-sensitive (
age
andAge
are different variables).Reserved keywords (like
if
,for
,print
, etc.) cannot be used as variable names.
Data Types in Python
Python offers a variety of built-in data types to handle different kinds of data. Let’s explore the primary data types and their uses.
1. Numeric Data Types
Numeric data types are used to store numbers and include:
Integer (
int
): Whole numbers (e.g.,10
,-5
).Float (
float
): Decimal numbers (e.g.,3.14
,-0.99
).Complex (
complex
): Numbers with real and imaginary parts (e.g.,3+5j
).
# Numeric types
a = 10 # Integer
b = 3.14 # Float
c = 2 + 3j # Complex
print(type(a)) # Output: <class 'int'>
print(type(b)) # Output: <class 'float'>
print(type(c)) # Output: <class 'complex'>
2. String (str
)
Strings are sequences of characters enclosed in either single or double quotes.
Example:# String examples
greeting = "Hello, World!"
char = 'A'
# String operations
print(greeting.upper()) # Output: HELLO, WORLD!
print(greeting.lower()) # Output: hello, world!
print(greeting[0:5]) # Output: Hello
3. Boolean (bool
)
Booleans represent one of two values: True
or False
.
# Boolean examples
is_python_easy = True
is_maths_hard = False
print(is_python_easy) # Output: True
print(type(is_python_easy)) # Output: <class 'bool'>
4. Sequence Types
Python includes three main sequence types:
List: Ordered, mutable collection.
Tuple: Ordered, immutable collection.
Range: Sequence of numbers.
fruits = ["apple", "banana", "cherry"]
print(fruits[0]) # Output: apple
fruits.append("orange") # Add an element
print(fruits) # Output: ['apple', 'banana', 'cherry', 'orange']
Tuple Example:
coordinates = (10, 20, 30)
print(coordinates[1]) # Output: 20
5. Dictionary (dict
)
Dictionaries store key-value pairs.
Example:student = {"name": "Alice", "age": 25, "grade": "A"}
print(student["name"]) # Output: Alice
Input and Output in Python
Input and output (I/O) operations allow you to interact with the user. Python provides built-in functions for this purpose.
1. Input in Python
The input()
function is used to take input from the user. By default, it reads the input as a string.
name = input("Enter your name: ")
print(f"Hello, {name}!")
Type Conversion for Numeric InputTo handle numeric input, you need to convert the string to the desired data type using int()
, float()
, etc.
age = int(input("Enter your age: "))
print(f"You are {age} years old.")
2. Output in Python
The print()
function is used to display output.
# Print with default behavior
print("Hello, Python!")
# Print with formatting
name = "Alice"
age = 25
print(f"My name is {name} and I am {age} years old.")
End and Separator Parametersend
Parameter: Changes the ending character (default is newline\n
).sep
Parameter: Changes the separator between items.
# Using end and sep
print("Hello", end="! ")
print("Welcome to Python.")
print("apple", "banana", "cherry", sep=", ")
Common Operations with Variables and Data Types
Here are some essential operations you can perform with Python’s data types:
1. Arithmetic Operations
x = 10
y = 3
print(x + y) # Addition: 13
print(x - y) # Subtraction: 7
print(x * y) # Multiplication: 30
print(x / y) # Division: 3.333...
print(x % y) # Modulus: 1
2. String Manipulation
text = "Python Basics"
print(text.lower()) # Output: python basics
print(text.upper()) # Output: PYTHON BASICS
print(text.replace("Basics", "Advanced")) # Output: Python Advanced
3. List Operations
numbers = [1, 2, 3, 4]
numbers.append(5)
print(numbers) # Output: [1, 2, 3, 4, 5]
numbers.pop(2) # Remove item at index 2
print(numbers) # Output: [1, 2, 4, 5]
Practical Examples of Variables, Data Types, and I/O
Here are a few practical examples that combine these concepts:
Example 1: Simple Calculator
# Take two numbers as input
num1 = float(input("Enter the first number: "))
num2 = float(input("Enter the second number: "))
# Perform addition
result = num1 + num2
print(f"The sum is: {result}")
Example 2: Personal Details
# Collect user details
name = input("Enter your name: ")
age = int(input("Enter your age: "))
# Display personalized message
print(f"Hi {name}, you are {age} years old!")
Conclusion
Understanding Python’s basics—variables, data types, and input/output—is crucial for any developer. These concepts form the foundation for more advanced topics like functions, loops, and data structures. Practice these basics thoroughly, experiment with the examples, and build simple programs to strengthen your understanding.
By mastering these fundamentals, you’ll be well on your way to becoming a proficient Python programmer.
Happy coding!