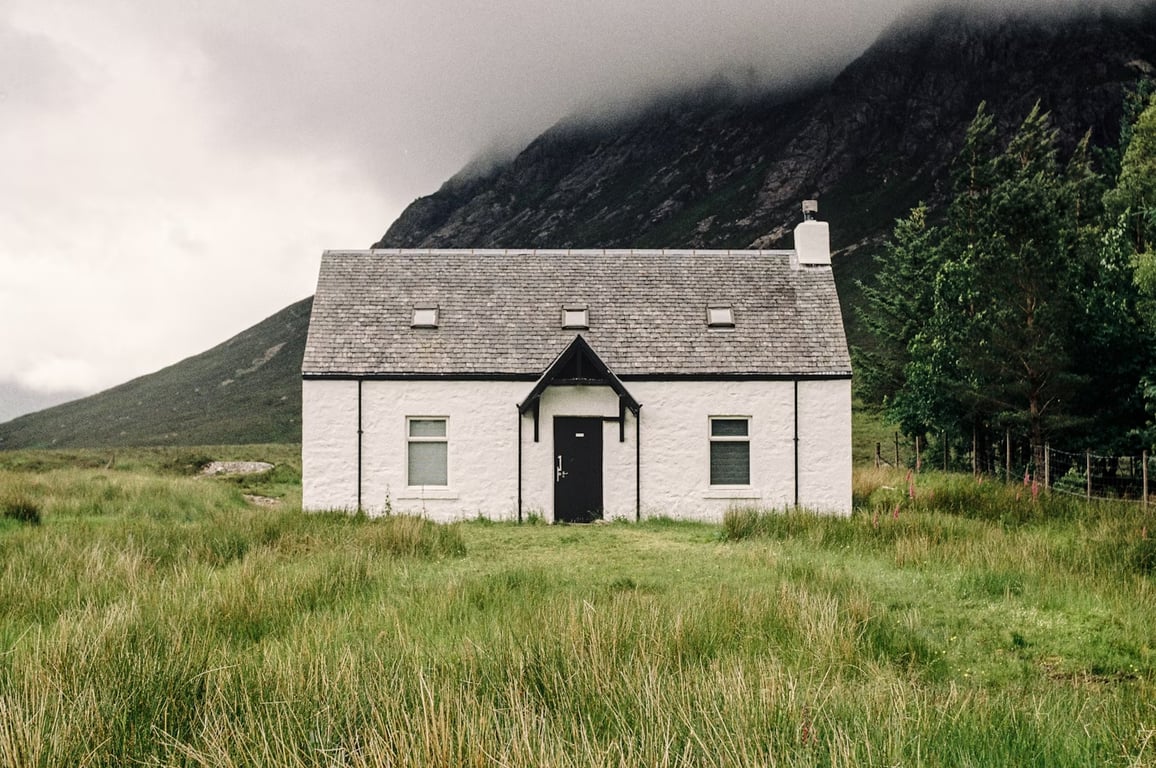
Photo by Alex Harwood on Unsplash
Object-Oriented Programming (OOP) is a programming paradigm that organizes code into reusable and modular structures called classes and objects. Python, being a versatile and high-level language, supports OOP and makes it easy to work with. Whether you’re a beginner or an experienced developer, understanding OOP is crucial for building complex, scalable, and efficient applications.
This blog will break down OOP concepts in Python with a focus on classes, objects, and related principles, simplifying them with real-world examples.
What is Object-Oriented Programming (OOP)?
OOP is a way of structuring a program by bundling related properties and behaviors into individual objects. It revolves around the following key concepts:
Classes: Blueprints for creating objects.
Objects: Instances of classes that encapsulate data and behaviors.
Inheritance: Mechanism for one class to inherit properties and methods of another.
Encapsulation: Hiding internal details and showing only necessary functionality.
Polymorphism: Defining methods in multiple ways for flexibility and reuse.
Why Use OOP in Python?
Modularity: Divides code into smaller, reusable components.
Scalability: Makes it easier to add new features without rewriting code.
Reusability: Promotes code reuse through inheritance and polymorphism.
Improved Maintenance: Simplifies debugging and updating.
Classes and Objects in Python
What is a Class?
A class is a template or blueprint for creating objects. It defines the properties (attributes) and actions (methods) that its objects will have.
What is an Object?
An object is an instance of a class. It is a real-world entity with attributes (data) and behaviors (methods).
Creating a Class
To define a class in Python, use the class
keyword.
Syntax:
class ClassName:
# Attributes and methods
pass
Example:
class Car:
# A simple class with an attribute and a method
def __init__(self, brand, model):
self.brand = brand
self.model = model
def display_info(self):
print(f"Car: {self.brand} {self.model}")
Here, __init__
is the constructor method that initializes the object’s attributes.
Creating an Object
To create an object, call the class like a function.
Example:
# Creating an object of the Car class
my_car = Car("Toyota", "Corolla")
# Accessing attributes and methods
print(my_car.brand) # Output: Toyota
my_car.display_info() # Output: Car: Toyota Corolla
Key OOP Principles in Python
1. Encapsulation
Encapsulation is the process of bundling data (attributes) and methods into a single unit (class) and restricting direct access to some components.
Example: Private Attributes
class BankAccount:
def __init__(self, owner, balance):
self.owner = owner
self.__balance = balance # Private attribute
def deposit(self, amount):
self.__balance += amount
def get_balance(self):
return self.__balance
# Creating an object
account = BankAccount("Alice", 1000)
account.deposit(500)
print(account.get_balance()) # Output: 1500
# Accessing private attribute (will raise an error)
# print(account.__balance) # AttributeError
2. Inheritance
Inheritance allows one class (child class) to inherit properties and methods from another (parent class).
Example:
class Vehicle:
def __init__(self, brand):
self.brand = brand
def start(self):
print(f"{self.brand} is starting.")
# Child class
class Car(Vehicle):
def __init__(self, brand, model):
super().__init__(brand)
self.model = model
def display_info(self):
print(f"Car: {self.brand} {self.model}")
# Creating an object of the child class
my_car = Car("Toyota", "Camry")
my_car.start() # Output: Toyota is starting.
my_car.display_info() # Output: Car: Toyota Camry
3. Polymorphism
Polymorphism allows methods to have different implementations in derived classes.
Example:
class Animal:
def sound(self):
pass
class Dog(Animal):
def sound(self):
print("Bark!")
class Cat(Animal):
def sound(self):
print("Meow!")
# Polymorphism in action
animals = [Dog(), Cat()]
for animal in animals:
animal.sound()
# Output:
# Bark!
# Meow!
4. Abstraction
Abstraction focuses on hiding implementation details and showing only essential features. In Python, abstraction can be implemented using abstract base classes (ABCs).
Example:
from abc import ABC, abstractmethod
class Shape(ABC):
@abstractmethod
def area(self):
pass
class Rectangle(Shape):
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
# Creating an object of the derived class
rect = Rectangle(5, 10)
print(rect.area()) # Output: 50
Real-World Examples of OOP in Python
1. Managing a Library System
class Book:
def __init__(self, title, author):
self.title = title
self.author = author
class Library:
def __init__(self):
self.books = []
def add_book(self, book):
self.books.append(book)
def display_books(self):
for book in self.books:
print(f"{book.title} by {book.author}")
# Adding and displaying books
library = Library()
library.add_book(Book("1984", "George Orwell"))
library.add_book(Book("To Kill a Mockingbird", "Harper Lee"))
library.display_books()
# Output:
# 1984 by George Orwell
# To Kill a Mockingbird by Harper Lee
2. Building an E-Commerce Application
class Product:
def __init__(self, name, price):
self.name = name
self.price = price
def display(self):
print(f"Product: {self.name}, Price: {self.price}")
class ShoppingCart:
def __init__(self):
self.items = []
def add_item(self, product):
self.items.append(product)
def total_price(self):
return sum(item.price for item in self.items)
# Adding products to the cart
cart = ShoppingCart()
cart.add_item(Product("Laptop", 1000))
cart.add_item(Product("Mouse", 50))
print(f"Total: {cart.total_price()}") # Output: Total: 1050
Advantages of OOP in Python
Code Reusability: Reuse code across multiple programs through inheritance.
Modular Design: Classes promote modularity, making debugging and maintenance easier.
Flexibility: Polymorphism enables flexibility in code execution.
Real-World Mapping: Models real-world scenarios effectively.
Common Mistakes to Avoid
Overusing Classes: Use OOP only when it fits the problem domain.
Ignoring Encapsulation: Avoid exposing internal details unnecessarily.
Creating Complex Hierarchies: Keep the inheritance tree shallow and simple.
Conclusion
Object-Oriented Programming in Python is a powerful paradigm that simplifies the design and implementation of scalable applications. By understanding the principles of classes, objects, inheritance, encapsulation, and polymorphism, you can write cleaner, reusable, and maintainable code.
Start practicing these OOP concepts in your Python projects, and soon, you’ll find them indispensable in tackling real-world programming challenges.
Happy coding!