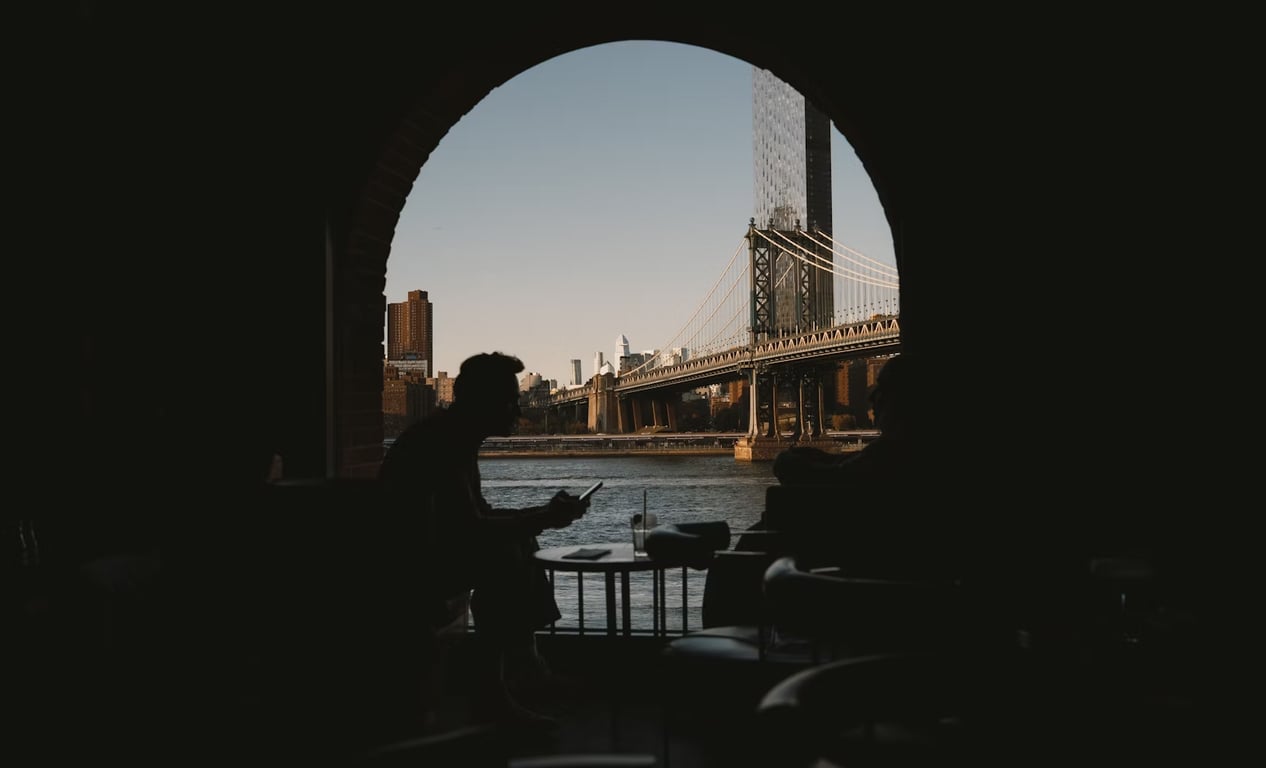
Python is a highly dynamic and flexible language, and one of its most powerful features is the ability to customize the behavior of classes and objects. While many Python developers are familiar with classes, instances, and inheritance, there is a more advanced concept that can be challenging to grasp: metaclasses.
Metaclasses in Python allow you to control the creation and behavior of classes themselves. In this blog, we will explore what metaclasses are, how they work, and when and why you might want to use them. By the end of this post, you'll have a solid understanding of Python's metaclass mechanism and how to leverage it in your own code.
What Are Metaclasses in Python?
In Python, everything is an object, including classes. Normally, when you define a class, Python uses a metaclass to create the class. In most cases, the default metaclass is type
, but metaclasses give you a way to customize the creation of classes themselves.
To understand metaclasses, it helps to first review how classes are created in Python. When you define a class, the process follows these steps:
The class body is executed. The class body is a block of code inside the
class
declaration. Any functions, variables, and other statements inside the class body are executed when the class is defined.The metaclass is determined. By default, the metaclass for a class is
type
. However, you can specify a different metaclass using themetaclass
keyword.The metaclass creates the class. The metaclass is responsible for creating the class itself. It is a class of a class.
A metaclass is essentially a "class factory." It defines how classes themselves behave. You can think of metaclasses as blueprints for classes, just as classes are blueprints for objects.
Metaclass Syntax
You can specify a metaclass using the metaclass
keyword in the class definition. Here's the basic syntax for defining a class with a custom metaclass:
class MyClass(metaclass=MyMeta):
pass
In this example, MyMeta
is a metaclass, and MyClass
is the class that will be created by MyMeta
.
How Do Metaclasses Work?
To better understand how metaclasses work, let’s go through an example that demonstrates their functionality.
Example 1: Default Metaclass (type)
By default, Python uses the type
metaclass to create classes. Here's a simple example:
class MyClass:
pass
print(type(MyClass)) # Output: <class 'type'>
In this case, type(MyClass)
returns <class 'type'>
, indicating that the class MyClass
is an instance of the type
metaclass. This is the default behavior in Python, but we can define our own custom metaclasses to change how classes behave.
Example 2: Creating a Custom Metaclass
Let’s now define a custom metaclass that modifies the behavior of classes during their creation. In this example, the metaclass will automatically add a method to any class that uses it.
class MyMeta(type):
def __new__(cls, name, bases, dct):
# Add a custom method to the class
dct['greet'] = lambda self: f"Hello from {self.__class__.__name__}"
return super().__new__(cls, name, bases, dct)
class MyClass(metaclass=MyMeta):
pass
obj = MyClass()
print(obj.greet()) # Output: Hello from MyClass
Explanation:
MyMeta
inherits fromtype
, making it a metaclass.The
__new__
method is overridden inMyMeta
. This method is called when a class is created. Inside__new__
, we add a method calledgreet
to the class's dictionary (dct
).When we create an instance of
MyClass
, thegreet
method is available, and callinggreet()
prints a message that includes the name of the class.
In this case, MyMeta
is a metaclass that adds functionality to classes at the time of their creation.
Metaclass Hooks
Metaclasses have several important hooks that allow you to control the class creation process. These hooks include:
__new__(cls, name, bases, dct)
: This is the primary hook used to create a new class. It is called when a class is being created, and it allows you to modify or replace the class before it is returned.__init__(cls, name, bases, dct)
: This hook is called after the class has been created, and it allows you to modify the class after it has been instantiated.__call__(cls, *args, **kwargs)
: This hook allows the metaclass to define custom behavior when a class is instantiated. For example, you could modify how objects of the class are created.
Example 3: Using __new__
and __init__
Let’s define a metaclass that uses both __new__
and __init__
hooks to modify a class:
class MyMeta(type):
def __new__(cls, name, bases, dct):
print(f"Creating class {name} with MyMeta")
return super().__new__(cls, name, bases, dct)
def __init__(cls, name, bases, dct):
print(f"Initializing class {name}")
super().__init__(name, bases, dct)
class MyClass(metaclass=MyMeta):
pass
Explanation:
__new__
is called when the class is created, and it allows us to intercept the class creation process.__init__
is called after the class is created and can be used to initialize any properties or perform additional actions.
When you run the code, the output will be:
Creating class MyClass with MyMeta
Initializing class MyClass
This shows how metaclasses can be used to hook into the class creation process.
When Should You Use Metaclasses?
Metaclasses are a powerful tool, but they are not something you should use unless you really need them. They introduce complexity, and in many cases, there are simpler and more readable ways to accomplish the same tasks. However, metaclasses can be extremely useful in certain scenarios.
Here are some cases where using a metaclass might be a good idea:
1. Enforcing Coding Standards or Design Patterns
Metaclasses can be used to enforce certain coding standards or design patterns across a set of classes. For example, you can create a metaclass that ensures all classes have certain methods or attributes.
class InterfaceMeta(type):
def __new__(cls, name, bases, dct):
if 'method' not in dct:
raise TypeError(f"Class {name} must implement 'method'")
return super().__new__(cls, name, bases, dct)
class MyClass(metaclass=InterfaceMeta):
def method(self):
pass # Implement method
# This will raise an error:
# class AnotherClass(metaclass=InterfaceMeta):
# pass # Missing method implementation
n this example, the InterfaceMeta
metaclass ensures that any class created with it must implement a method
function. This can be used to enforce interface-like behavior.
2. Automatically Adding Methods or Attributes
As shown in previous examples, metaclasses allow you to automatically add methods or attributes to classes. This can be useful when you want all your classes to have the same set of behaviors.
class AddTimestampMeta(type):
def __new__(cls, name, bases, dct):
dct['timestamp'] = "2025-01-01"
return super().__new__(cls, name, bases, dct)
class MyClass(metaclass=AddTimestampMeta):
pass
print(MyClass.timestamp) # Output: 2025-01-01
This example shows how to use a metaclass to add a timestamp
attribute to all classes that use it.
3. Logging or Debugging Class Creation
Metaclasses can also be used to log or debug the class creation process. For example, you can print debug information every time a class is created or modified.
class LoggingMeta(type):
def __new__(cls, name, bases, dct):
print(f"Creating class {name}")
return super().__new__(cls, name, bases, dct)
class MyClass(metaclass=LoggingMeta):
pass
In this case, the LoggingMeta
metaclass prints a message whenever a class is created.
4. Extending Python’s Object-Oriented Features
Metaclasses allow you to extend Python’s object-oriented capabilities. You can modify the behavior of classes, control their instantiation, and even build your own object-oriented frameworks by using metaclasses.
Conclusion
Metaclasses are an advanced feature of Python that gives you full control over how classes are created and behave. By customizing metaclasses, you can enforce coding standards, automatically add attributes and methods, log class creation, and more. However, because they add complexity to the code, metaclasses should only be used when necessary.
In this blog, we’ve covered:
What metaclasses are and how they work.
How to create custom metaclasses using
__new__
and__init__
.When and why to use metaclasses in real-world Python projects.
Metaclasses are a powerful tool that, when used correctly, can enhance your ability to write reusable, flexible, and maintainable code. Understanding when to use them and how to implement them effectively is crucial for writing more advanced Python applications.
Happy coding!