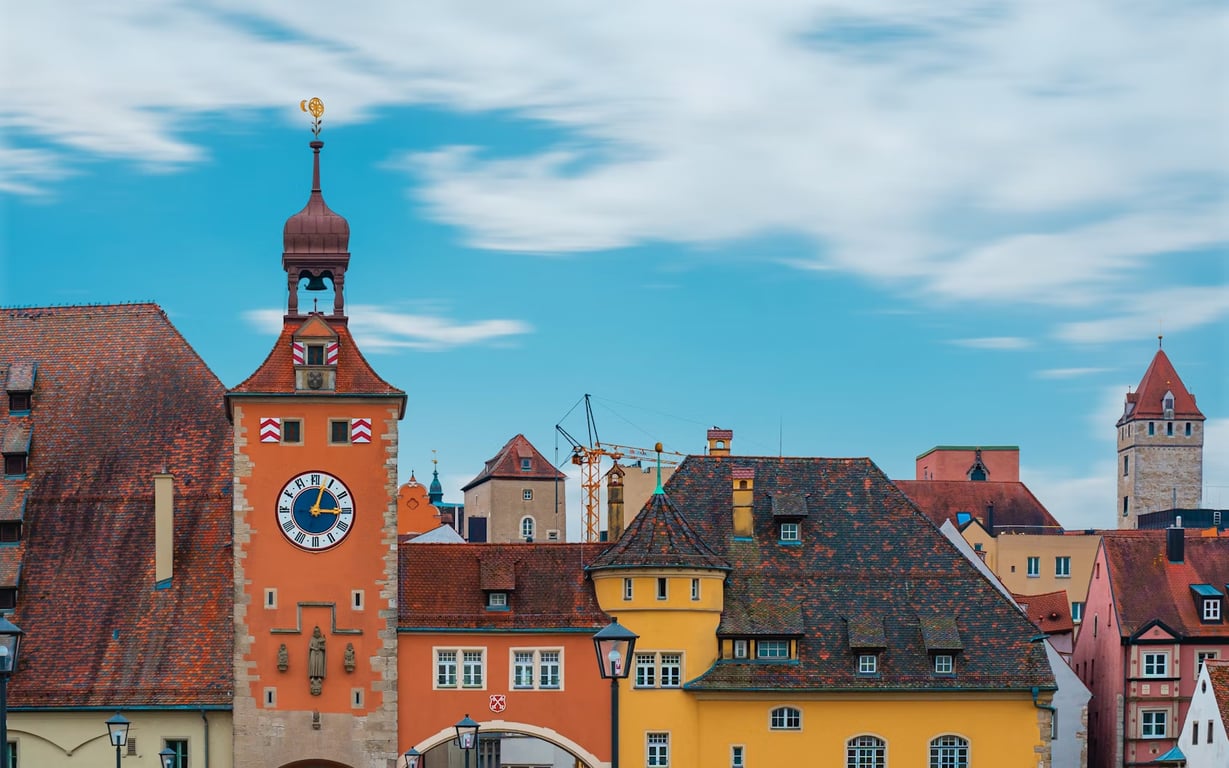
Photo by Jahanzeb Ahsan on Unsplash
Python’s ease of use, coupled with its flexibility and power, has made it one of the most popular programming languages in the world. One of Python's strongest features is its modular design, which encourages the creation and reuse of code in the form of modules and packages. This modular approach allows developers to keep their codebase clean, maintainable, and scalable.
In this blog, we’ll dive into how to build custom Python modules and packages. By the end, you’ll be able to write your own reusable Python code and share it with others or use it across multiple projects.
What Are Python Modules and Packages?
Python Modules
In Python, a module is simply a file containing Python code. A module can define functions, classes, and variables that you can include in your application. Essentially, modules are just Python files with the .py
extension that can be imported into other Python programs.
You can think of a module as a toolbox. Whenever you need a specific tool (like a function or a variable) from that toolbox, you can import it into your program.
Example of a ModuleLet's create a simple Python module called math_tools.py
that contains some mathematical functions.
# math_tools.py
def add(a, b):
"""Return the sum of two numbers."""
return a + b
def multiply(a, b):
"""Return the product of two numbers."""
return a * b
This math_tools.py
file is a Python module, and you can now use it in any other Python program by importing it.
# main.py
import math_tools
result_add = math_tools.add(5, 10)
result_multiply = math_tools.multiply(5, 10)
print(f"Addition: {result_add}")
print(f"Multiplication: {result_multiply}")
Here, we imported the math_tools
module into the main.py
file, then used its functions add()
and multiply()
to perform operations.
Python Packages
A Python package is a collection of modules grouped together in a directory. A package can contain multiple modules, sub-packages, and a special __init__.py
file that allows the Python interpreter to recognize the directory as a package.
A package is essentially a way to organize related modules, making it easier to distribute and manage your code.
Structure of a Python PackageHere’s what a basic package structure might look like:
my_package/
__init__.py
module1.py
module2.py
sub_package/
__init__.py
module3.py
my_package/
: The main package directory.__init__.py
: This special file indicates that the directory should be treated as a package. It can be empty or contain package initialization code.module1.py
,module2.py
: These are individual modules within the package.sub_package/
: A sub-package containing its own modules and an__init__.py
file.
Creating a Simple Custom Python Module
Let’s walk through the steps of creating your own Python module.
Step 1: Create Your Module File
First, create a new directory for your project if you don’t already have one. Inside this directory, create a Python file that will be your module. For this example, let's create a file named greeting.py
.
# greeting.py
def greet(name):
"""Return a greeting message for the given name."""
return f"Hello, {name}!"
Step 2: Use Your Module
Now that you’ve created your greeting.py
module, you can import and use it in another Python script.
# main.py
import greeting
message = greeting.greet("Alice")
print(message) # Output: Hello, Alice!
Step 3: Organizing Code into Multiple Functions
A module can have multiple functions, variables, and classes. Here’s how you might expand the greeting.py
module by adding more functionality.
# greeting.py
def greet(name):
"""Return a greeting message for the given name."""
return f"Hello, {name}!"
def farewell(name):
"""Return a farewell message for the given name."""
return f"Goodbye, {name}!"
You can now call both greet()
and farewell()
from the greeting
module in your main.py
file.
# main.py
import greeting
print(greeting.greet("Alice"))
print(greeting.farewell("Alice"))
Step 4: Making Your Module More Reusable
In Python, you can make your code more reusable by allowing users to run your module as a script, as well as importing it into other programs.
Here’s an example of how to add a check to ensure your module can be run directly or imported:
# greeting.py
def greet(name):
"""Return a greeting message for the given name."""
return f"Hello, {name}!"
def farewell(name):
"""Return a farewell message for the given name."""
return f"Goodbye, {name}!"
if __name__ == "__main__":
# Code to execute when the module is run as a script
name = input("Enter your name: ")
print(greet(name))
print(farewell(name))
With this modification, you can now run the greeting.py
module directly and interact with it, or import it into another script.
Creating a Python Package
Now, let's take things a step further and create a Python package.
Step 1: Package Structure
Start by organizing your code into a package directory. For example, let’s create a package called mytools
with two modules: math_tools.py
and string_tools.py
.
mytools/
__init__.py
math_tools.py
string_tools.py
__init__.py
is the initialization file for the package, and it can be left empty for now.math_tools.py
will contain some mathematical functions, whilestring_tools.py
will contain string manipulation functions.
Step 2: Write Code for Each Module
math_tools.py# math_tools.py
def add(a, b):
"""Return the sum of two numbers."""
return a + b
def subtract(a, b):
"""Return the difference between two numbers."""
return a - b
string_tools.py
# string_tools.py
def to_uppercase(text):
"""Convert a string to uppercase."""
return text.upper()
def to_lowercase(text):
"""Convert a string to lowercase."""
return text.lower()
Step 3: Initialize the Package
In the __init__.py
file, you can import the modules so that they’re easily accessible when the package is imported.
# __init__.py
from .math_tools import add, subtract
from .string_tools import to_uppercase, to_lowercase
Now, you’ve created a package mytools
that includes two modules (math_tools
and string_tools
) and a convenient initialization file.
Step 4: Use Your Package
You can now import your package and use the functions defined within it.
# main.py
import mytools
# Using math tools
print(mytools.add(5, 3))
print(mytools.subtract(10, 4))
# Using string tools
print(mytools.to_uppercase("hello"))
print(mytools.to_lowercase("WORLD"))
This structure is very scalable. You can add more modules, sub-packages, and functions as your package grows, keeping your code organized and easy to manage.
Distributing Your Python Module or Package
After building a custom Python module or package, you might want to share it with others. Here’s how you can distribute your code:
1. Create a setup.py
File
If you’re planning to distribute your package through the Python Package Index (PyPI), you’ll need to create a setup.py
file. This file contains metadata about your package, such as its name, version, description, and dependencies.
# setup.py
from setuptools import setup, find_packages
setup(
name="mytools",
version="0.1",
packages=find_packages(),
description="A collection of useful tools for mathematical and string operations.",
author="Your Name",
author_email="your.email@example.com",
url="https://github.com/yourusername/mytools",
)
2. Upload to PyPI
Once you’ve created your package and setup.py
file, you can use twine
to upload it to PyPI. First, make sure you have twine
installed:
pip install twine
Next, build your package and upload it:
python setup.py sdist
twine upload dist/*
Your package is now available for installation via pip!
Conclusion
Building custom Python modules and packages is a great way to organize and reuse your code. Whether you’re working on a personal project or planning to distribute your code to the Python community, knowing how to create and structure your modules and packages is an essential skill.
In this blog, we covered:
How to create and use Python modules.
The structure of a Python package.
Organizing code into reusable components.
How to distribute your Python package using
setup.py
.
By following these steps, you can write clean, maintainable code that you can reuse across different projects or share with others in the Python community.
Happy coding!