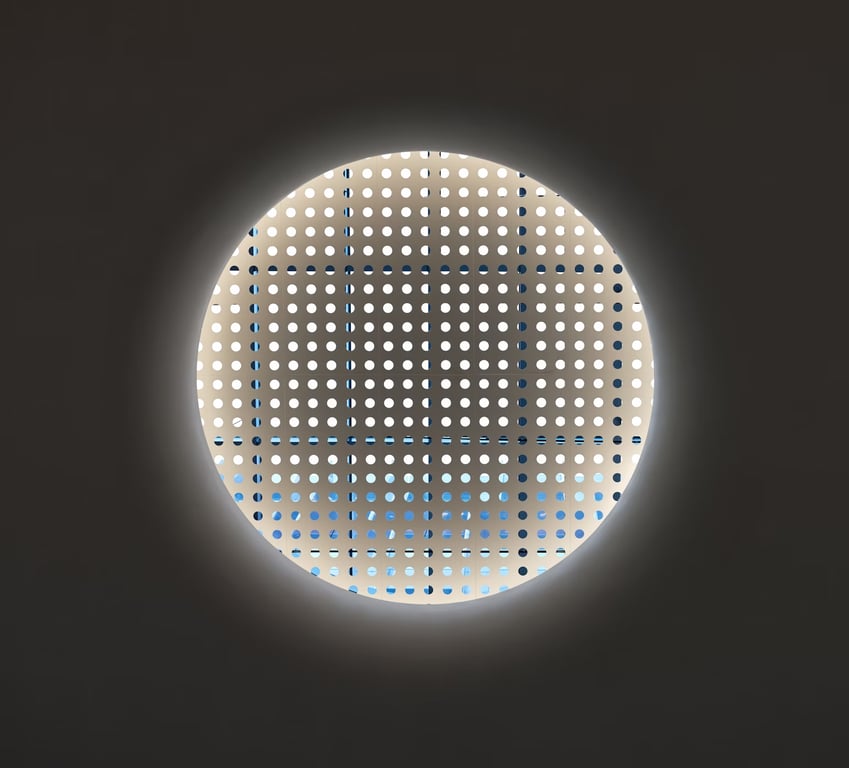
Photo by Declan Sun on Unsplash
File system operations are at the core of many programming tasks, from managing files and directories to automating system tasks. Python offers two powerful modules, os
and shutil
, to help you interact with the file system efficiently. These modules provide robust tools for handling files, directories, and paths, making them indispensable for any Python developer.
In this blog, we’ll explore the os
and shutil
modules in-depth, covering their features, use cases, and practical examples. By the end, you'll have a solid grasp of these modules and their capabilities.
Why Learn os and shutil Modules?
The os
module provides functions to interact with the operating system, such as reading or writing to the file system, handling environment variables, and working with processes. The shutil
module complements it by offering high-level operations for copying, moving, and removing files and directories.
Key Features of os
and shutil
:
File and directory management.
Path handling and traversal.
Environment variable access.
File copying, moving, and removal.
Directory archiving and compression.
Setting Up
Before diving into examples, ensure you have Python installed on your system. The os
and shutil
modules are part of Python’s standard library, so no additional installation is required.
1. File and Directory Operations with the os Module
The os
module provides tools to interact with the file system directly.
Checking the Current Working Directory
The current working directory is the folder where your Python script is running.
import os
# Get the current working directory
cwd = os.getcwd()
print(f"Current working directory: {cwd}")
Changing the Current Directory
Use os.chdir()
to change the working directory.
# Change the current working directory
os.chdir("/path/to/new/directory")
print(f"Changed working directory: {os.getcwd()}")
Listing Directory Contents
To view the contents of a directory, use os.listdir()
.
# List files and directories
files = os.listdir(".")
print("Contents of the directory:", files)
Creating and Removing Directories
Create directories using os.mkdir()
and os.makedirs()
. Remove them using os.rmdir()
.
# Create a single directory
os.mkdir("test_dir")
# Create nested directories
os.makedirs("parent_dir/child_dir")
# Remove a single directory
os.rmdir("test_dir")
# Remove nested directories
os.removedirs("parent_dir/child_dir")
2. File Path Handling with os.path
The os.path
module offers functions to manipulate file and directory paths.
Checking Path Existence
Use os.path.exists()
to check if a file or directory exists.
file_path = "example.txt"
if os.path.exists(file_path):
print(f"{file_path} exists.")
else:
print(f"{file_path} does not exist.")
oining Paths
Combine directory and file names into a complete path using os.path.join()
.
dir_name = "/home/user"
file_name = "document.txt"
full_path = os.path.join(dir_name, file_name)
print(f"Full path: {full_path}")
Splitting Paths
Split a path into its directory and file components with os.path.split()
.
path = "/home/user/document.txt"
directory, file = os.path.split(path)
print(f"Directory: {directory}, File: {file}")
3. High-Level File Operations with shutil
The shutil
module simplifies complex file and directory operations.
Copying Files
Use shutil.copy()
or shutil.copy2()
to copy files. The latter also preserves metadata.
import shutil
# Copy a file
shutil.copy("source.txt", "destination.txt")
# Copy a file with metadata
shutil.copy2("source.txt", "destination.txt")
Copying Directories
Use shutil.copytree()
to copy an entire directory.
# Copy a directory
shutil.copytree("source_dir", "destination_dir")
Moving Files and Directories
Move files or directories with shutil.move()
.
# Move a file
shutil.move("source.txt", "new_folder/source.txt")
Removing Files and Directories
Use shutil.rmtree()
to remove directories recursively.
# Remove a directory and its contents
shutil.rmtree("old_directory")
4. Archiving and Compression
The shutil
module can also create and extract archives.
Creating an Archive
Use shutil.make_archive()
to create zip or tar archives.
# Create a zip archive
shutil.make_archive("backup", "zip", "source_directory")
Extracting an Archive
Although shutil
does not extract archives, you can use the zipfile
or tarfile
modules for this purpose.
5. Combining os and shutil for Advanced Tasks
By combining the capabilities of os
and shutil
, you can create robust scripts for file system automation.
Example: Organizing Files by Extension
import os
import shutil
def organize_files_by_extension(folder_path):
for file_name in os.listdir(folder_path):
full_path = os.path.join(folder_path, file_name)
if os.path.isfile(full_path):
# Get file extension
_, ext = os.path.splitext(file_name)
ext = ext[1:] # Remove leading dot
if ext:
# Create a folder for the extension if it doesn't exist
ext_folder = os.path.join(folder_path, ext)
os.makedirs(ext_folder, exist_ok=True)
# Move the file
shutil.move(full_path, os.path.join(ext_folder, file_name))
organize_files_by_extension("downloads")
6. Handling Errors and Exceptions
File system operations can fail due to permissions, missing files, or other issues. Handle these errors gracefully using try-except
blocks.
Example: Safely Removing a Directory
try:
shutil.rmtree("non_existent_dir")
except FileNotFoundError:
print("Directory does not exist.")
except PermissionError:
print("Permission denied.")
7. Best Practices for File System Operations
Use Absolute Paths: Avoid relative paths to prevent ambiguity.
Handle Errors Gracefully: Anticipate issues like missing files or permission errors.
Test on Sample Data: Avoid running destructive operations (e.g., deletion) without testing.
Respect Permissions: Ensure you have the necessary permissions before modifying files or directories.
Backup Important Data: Always back up data before performing operations that might lead to data loss.
8. Real-World Use Cases
1. Cleaning Up Temporary Files
import tempfile
# Create and clean up temporary files
temp_file = tempfile.NamedTemporaryFile(delete=False)
print(f"Temporary file created: {temp_file.name}")
os.unlink(temp_file.name)
print("Temporary file deleted.")
2. Automating Backups
def backup_directory(source_dir, backup_dir):
shutil.copytree(source_dir, backup_dir)
print(f"Backup of {source_dir} created at {backup_dir}")
backup_directory("projects", "projects_backup")
Conclusion
Mastering Python’s os
and shutil
modules empowers you to perform complex file system operations effortlessly. Whether you’re managing directories, automating backups, or organizing files, these modules provide the tools you need. By following best practices and handling errors gracefully, you can build robust scripts that streamline your workflow.
Now that you’re equipped with these skills, start experimenting with your file system tasks.
Happy coding!