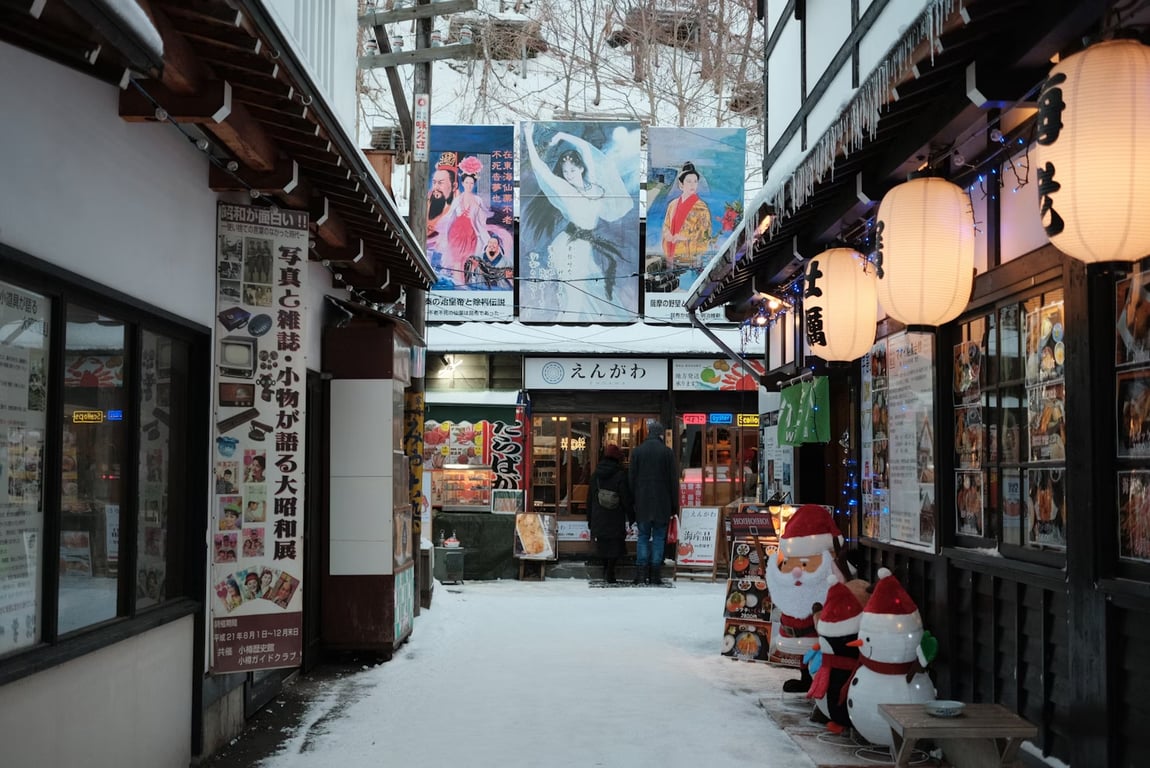
Photo by Dominic Kurniawan Suryaputra on Unsplash
Handling files is an essential task in any programming language. Whether you need to read user data from a file, process logs, or write information into a file for future reference, file handling is an integral part of software development. Python makes file handling incredibly easy with its built-in capabilities, allowing you to read and write files efficiently and safely.
In this blog, we’ll explore how to work with files in Python, covering essential concepts like opening, reading, writing, and closing files. We will also discuss various file modes, file handling techniques, and best practices for error handling. By the end of this guide, you’ll be equipped with the knowledge to work with files like a pro.
What is File Handling?
File handling refers to the operations you can perform on files, such as reading from and writing to them. Files can be either text files or binary files. Python provides built-in functions and methods to handle file operations seamlessly. It allows you to perform operations like opening, reading, writing, and closing files, all with minimal effort.
How to Open a File in Python
Before reading from or writing to a file, you must first open it. Python provides a built-in open()
function to open a file. The function takes two main arguments:
File Name: The name of the file you want to open (including the file extension).
Mode: The mode in which you want to open the file. The mode determines whether the file is being opened for reading, writing, appending, etc.
File Modes
Here are the most commonly used file modes in Python:
'r'
: Read (default mode). Opens the file for reading only. If the file doesn’t exist, it raises aFileNotFoundError
.'w'
: Write. Opens the file for writing. If the file exists, it truncates the file to zero length; otherwise, it creates a new file.'a'
: Append. Opens the file for appending. If the file doesn’t exist, it creates a new file.'rb'
: Read in binary mode.'wb'
: Write in binary mode.'r+'
: Read and write. Opens the file for both reading and writing.'w+'
: Write and read. Opens the file for both reading and writing, truncating the file to zero length if it exists.
Opening a File
Here’s an example of how to open a file in read mode:
file = open("example.txt", "r")
To open a file in write mode:
file = open("example.txt", "w")
Reading from Files
Once a file is opened in read mode ('r'
), you can read the contents of the file using several methods provided by the file object.
1. Using read()
The read()
method reads the entire file into a string. It is useful when the file is small and you want to process all its content at once.
file = open("example.txt", "r")
content = file.read()
print(content)
file.close()
2. Using readline()
The readline()
method reads one line at a time. This is ideal if the file is large, and you want to process it line by line.
file = open("example.txt", "r")
line1 = file.readline()
print(line1)
file.close()
3. Using readlines()
The readlines()
method reads the entire file into a list, where each line is a separate list element. This is useful when you want to work with individual lines after reading the file.
file = open("example.txt", "r")
lines = file.readlines()
for line in lines:
print(line)
file.close()
Writing to Files
In Python, writing to a file is done using the write()
or writelines()
methods. You need to open the file in write ('w'
) or append ('a'
) mode to write data to it.
1. Using write()
The write()
method writes a string to the file. If the file already contains data, the method overwrites it unless you open the file in append mode ('a'
).
file = open("example.txt", "w")
file.write("Hello, World!")
file.close()
2. Using writelines()
The writelines()
method writes a list of strings to the file. This is useful when you have multiple lines of text to write.
lines = ["First line\n", "Second line\n", "Third line\n"]
file = open("example.txt", "w")
file.writelines(lines)
file.close()
Appending to Files
If you want to add data to the end of an existing file without overwriting the existing content, you can use the append mode ('a'
). This ensures that the new data is added after the existing content.
file = open("example.txt", "a")
file.write("\nThis is an appended line.")
file.close()
Closing a File
It’s important to close a file after completing the read or write operation to free up system resources. You can close a file using the close()
method.
file = open("example.txt", "r")
content = file.read()
file.close()
Alternatively, Python provides a more elegant way of handling files using the with
statement, which automatically closes the file after the block of code is executed.
with open("example.txt", "r") as file:
content = file.read()
print(content)
The with
statement ensures that the file is closed even if an error occurs during the file operation, making it a safer way to work with files.
Error Handling in File Operations
When working with files, there’s always a chance that something might go wrong, such as trying to open a non-existent file or encountering a permission error. Python provides the try-except
block to handle errors gracefully.
Handling File Not Found Error
try:
file = open("non_existent_file.txt", "r")
except FileNotFoundError:
print("The file you are trying to open does not exist.")
Handling Permission Error
try:
file = open("example.txt", "w")
except PermissionError:
print("You do not have permission to write to this file.")
Reading and Writing Binary Files
While reading and writing text files is straightforward, binary files require a different approach. For binary files (like images, audio files, etc.), you need to open the file in binary mode using 'rb'
for reading and 'wb'
for writing.
Reading a Binary File
with open("image.jpg", "rb") as file:
binary_data = file.read()
print(binary_data[:10]) # Display the first 10 bytes of the file
Writing to a Binary File
with open("copy_image.jpg", "wb") as file:
file.write(binary_data)
Working with CSV Files
CSV (Comma Separated Values) files are widely used to store tabular data. Python provides a built-in csv
module to handle reading and writing CSV files.
Reading from a CSV File
import csv
with open("data.csv", "r") as file:
reader = csv.reader(file)
for row in reader:
print(row)
Writing to a CSV File
import csv
data = [["Name", "Age"], ["Alice", 30], ["Bob", 25]]
with open("data.csv", "w", newline="") as file:
writer = csv.writer(file)
writer.writerows(data)
Best Practices for File Handling in Python
Use
with
statement: It ensures that files are closed properly even if an error occurs during operations.Check for file existence: Always check if the file exists before reading, or handle exceptions gracefully if it doesn’t.
Ensure the correct file mode: Always ensure that you open the file in the correct mode (
'r'
,'w'
,'a'
, etc.).Close files: Always close files after operations are complete to avoid memory leaks.
Conclusion
Python’s file handling capabilities are robust and easy to use, making it an essential skill for every Python programmer. By mastering how to read from and write to files, handle errors, and work with different file formats, you will be able to efficiently manage data in your applications. The flexibility of file modes and error handling mechanisms ensures that file operations can be done safely and with minimal complexity.
Whether you’re working with simple text files, binary files, or complex formats like CSV, Python’s file handling features will help you efficiently tackle file manipulation tasks. With this knowledge, you’ll be well on your way to writing clean, reliable, and efficient code for handling files like a pro!
Happy coding!