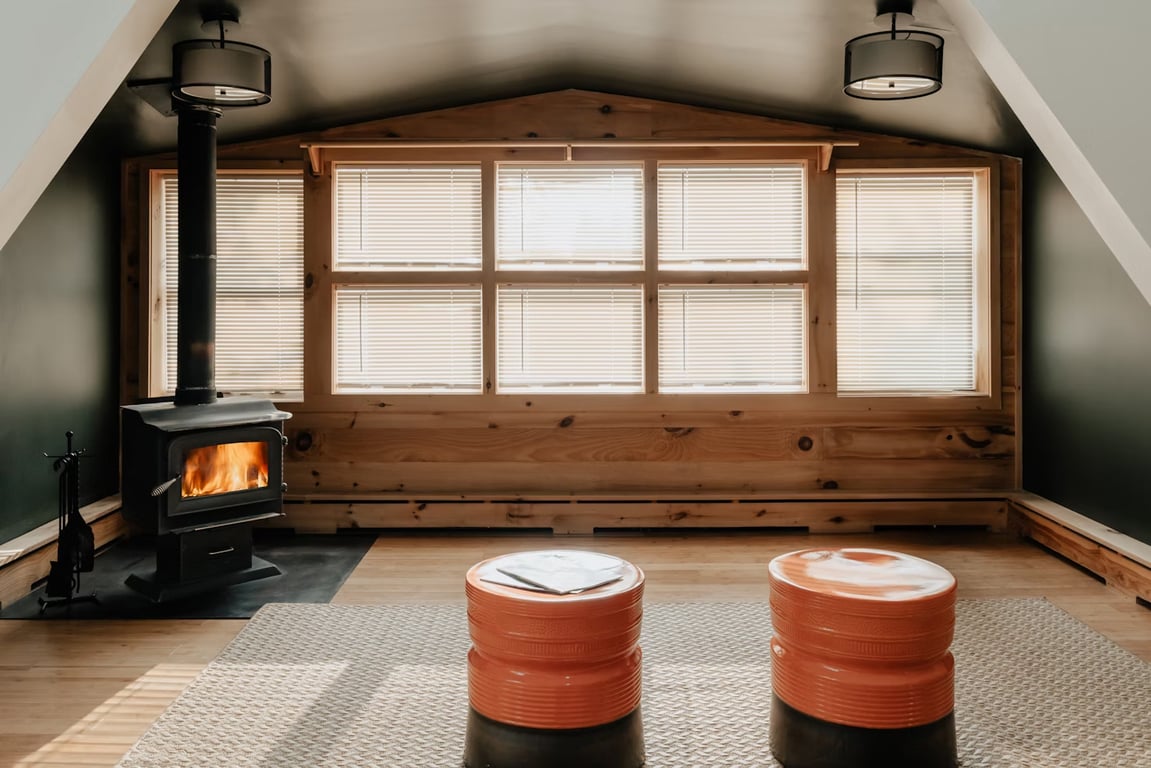
Photo by Clay Banks on Unsplash
Python is one of the most popular programming languages, and one of the key reasons for its success is its ability to write modular, reusable, and maintainable code. At the heart of this capability lies functions, which are essential for structuring code in a logical and organized way.
In this blog, we’ll explore everything about Python functions, from the basics to advanced techniques, with actionable tips and examples for writing clean and reusable code.
What Are Functions in Python?
A function is a block of code that performs a specific task. Functions help in breaking down complex problems into smaller, manageable chunks and allow code reuse, reducing redundancy.
Basic Syntax of a Function
def function_name(parameters):
# Code block
return value
Example: A Simple Function
def greet(name):
return f"Hello, {name}!"
print(greet("Alice")) # Output: Hello, Alice!
Why Use Functions?
1. Code Reusability
Functions let you write code once and use it multiple times, saving time and effort.
2. Improved Readability
Breaking a large task into smaller, logically named functions makes code more readable.
3. Ease of Maintenance
If a bug exists, you only need to fix it in one place.
4. Better Collaboration
Functions make it easier for teams to work on different parts of a program.
Types of Functions in Python
Python provides several types of functions based on how and where they are used:
1. Built-in Functions
These are predefined functions in Python, such as print()
, len()
, and sum()
.
2. User-Defined Functions
Functions that you create to perform specific tasks.
3. Lambda Functions
Anonymous, single-expression functions useful for short tasks.
4. Recursive Functions
Functions that call themselves to solve smaller instances of a problem.
Tips for Writing Clean and Reusable Functions
1. Use Meaningful Names
Choose descriptive and meaningful names for functions that clearly indicate their purpose.
Example:
# Poor naming
def func(a, b):
return a + b
# Good naming
def add_numbers(num1, num2):
return num1 + num2
2. Keep Functions Small and Focused
A function should ideally perform one task. If a function becomes too large or handles multiple responsibilities, consider breaking it into smaller functions.
Example:
# Large function (hard to maintain)
def process_data(data):
cleaned_data = [item.strip() for item in data]
sorted_data = sorted(cleaned_data)
return sorted_data
# Refactored into smaller functions
def clean_data(data):
return [item.strip() for item in data]
def sort_data(data):
return sorted(data)
def process_data(data):
return sort_data(clean_data(data))
3. Avoid Hardcoding Values
Use parameters to make your functions flexible and reusable.
Example:
# Hardcoded function
def calculate_discount():
price = 100
discount = 10
return price - discount
# Flexible function
def calculate_discount(price, discount):
return price - discount
4. Use Default Parameters
Default parameters allow you to set fallback values, making the function easier to use.
Example:
def greet(name="Guest"):
return f"Hello, {name}!"
print(greet()) # Output: Hello, Guest!
print(greet("Alice")) # Output: Hello, Alice!
5. Leverage Keyword Arguments
Keyword arguments improve the readability of function calls, especially for functions with multiple parameters.
Example:
def create_user(username, email, is_admin=False):
return f"User: {username}, Admin: {is_admin}"
# Using positional arguments
print(create_user("john_doe", "john@example.com"))
# Using keyword arguments
print(create_user(username="john_doe", email="john@example.com", is_admin=True))
6. Use Docstrings for Documentation
Document your functions with docstrings to describe their purpose, parameters, and return values.
Example:
def add_numbers(a, b):
"""
Adds two numbers and returns the result.
Parameters:
a (int): First number
b (int): Second number
Returns:
int: Sum of the two numbers
"""
return a + b
7. Avoid Side Effects
Functions should ideally not modify variables outside their scope. Use return values to pass data instead.
Example:
# Function with side effects
result = 0
def add_to_result(value):
global result
result += value
# Pure function
def add(a, b):
return a + b
Advanced Techniques for Writing Better Functions
**1. Use *args and kwargs for Flexibility
*args
lets you pass a variable number of positional arguments, and **kwargs
handles keyword arguments.
Example:
def greet_all(*names):
for name in names:
print(f"Hello, {name}!")
greet_all("Alice", "Bob", "Charlie")
# Output:
# Hello, Alice!
# Hello, Bob!
# Hello, Charlie!
def display_info(**details):
for key, value in details.items():
print(f"{key}: {value}")
display_info(name="Alice", age=30, city="New York")
# Output:
# name: Alice
# age: 30
# city: New York
2. Use Lambda Functions for Short Tasks
Lambda functions are useful for small, single-expression operations.
Example:
# Regular function
def square(x):
return x**2
# Lambda function
square = lambda x: x**2
print(square(5)) # Output: 25
3. Use Type Annotations
Type annotations make your code more readable and help prevent bugs.
Example:
def add_numbers(a: int, b: int) -> int:
return a + b
print(add_numbers(3, 4)) # Output: 7
4. Apply Recursive Functions for Repetitive Tasks
Use recursion to solve problems that can be broken into smaller sub-problems.
Example:
def factorial(n):
if n == 0:
return 1
return n * factorial(n - 1)
print(factorial(5)) # Output: 120
5. Avoid Overengineering
Keep your functions as simple as possible. Avoid adding unnecessary complexity.
Example:
# Overengineered
def is_even(number):
return True if number % 2 == 0 else False
# Simple and clean
def is_even(number):
return number % 2 == 0
Real-World Examples of Functions
1. Validating User Input
def validate_age(age):
if age < 0:
raise ValueError("Age cannot be negative")
return age
try:
print(validate_age(25)) # Output: 25
print(validate_age(-5)) # Raises ValueError
except ValueError as e:
print(e)
2. Sending Email Notifications
def send_email(to, subject, body):
print(f"Sending email to {to}")
print(f"Subject: {subject}")
print(f"Body: {body}")
send_email("user@example.com", "Welcome!", "Thank you for signing up.")
3. Generating Fibonacci Sequence
def fibonacci(n):
sequence = [0, 1]
for _ in range(2, n):
sequence.append(sequence[-1] + sequence[-2])
return sequence
print(fibonacci(10))
# Output: [0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
Best Practices for Function Design
Write Unit Tests: Test your functions to ensure they work as expected.
Follow DRY Principle: Don’t Repeat Yourself; reuse functions whenever possible.
Avoid Deep Nesting: Simplify logic to reduce deeply nested conditions.
Return Early: Exit functions as soon as the result is known to improve readability.
Conclusion
Functions are the backbone of Python programming. They allow you to write modular, clean, and reusable code, which is essential for creating scalable applications. By following the tips and techniques outlined in this blog, you can master the art of writing efficient Python functions that are easy to maintain and understand.
Start practicing these tips today and see how they transform your coding journey. Happy coding!