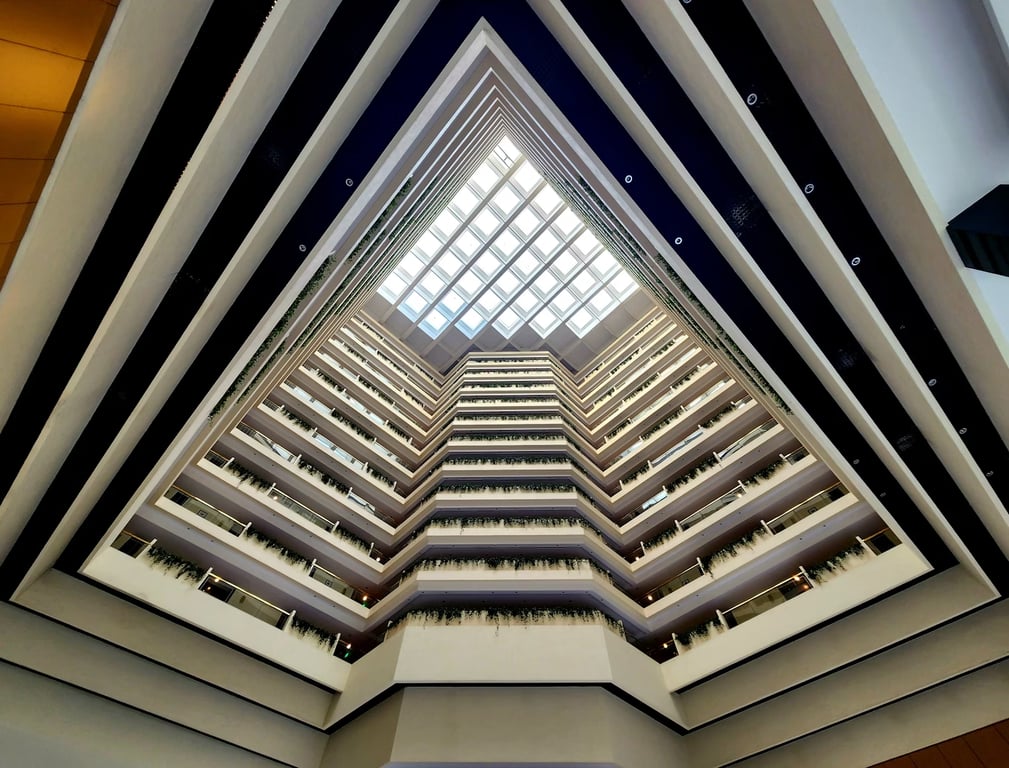
Photo by Zoshua Colah on Unsplash
Python, known for its simplicity and readability, is an excellent choice for anyone looking to dive into programming. Whether you’re a beginner with no coding experience or someone transitioning from another language, Python’s straightforward syntax makes it easy to learn.
In this blog, you’ll learn how to set up your Python environment, write your first program, and understand its key components—all in under 10 minutes. Let’s get started!
Why Python?
Before we dive in, let’s briefly understand why Python is so popular:
Beginner-Friendly: Python’s syntax is close to natural language, making it easier to understand.
Versatile: Used in web development, data science, machine learning, and more.
Large Community: Tons of resources, libraries, and tutorials are available to help you.
Cross-Platform: Python runs on Windows, macOS, and Linux.
Step 1: Setting Up Your Python Environment
1. Install Python
Python is free to download and install. Follow these steps:
Windows:
Visit python.org.
Download the latest Python version for Windows.
Run the installer and check the box labeled Add Python to PATH before installing.
macOS/Linux: Python comes pre-installed on most macOS/Linux systems. To check, open a terminal and type:
python3 --version
If not installed, you can use a package manager like
brew
on macOS orapt
on Linux.
2. Choose an Editor
To write your Python code, you need a text editor or an Integrated Development Environment (IDE). Here are some options:
Beginners: Use IDLE (comes pre-installed with Python).
Advanced Options: Visual Studio Code, PyCharm, or Jupyter Notebook.
3. Verify Installation
Once Python is installed, verify it by typing the following command in your terminal or command prompt:
python --version
or
python3 --version
This will display the installed Python version.
Step 2: Writing Your First Python Program
With your setup ready, let’s jump into coding.
1. Open Your Text Editor or IDE
If using IDLE, open it from your applications menu.
For Visual Studio Code, create a new file with the extension
.py
(e.g.,first_program.py
).
2. Write the Program
Let’s write the classic "Hello, World!" program. This program outputs a simple message to the screen.
# Your first Python program
print("Hello, World!")
Explanation:
The
print()
function displays the message inside the parentheses.Double or single quotes (
" "
or' '
) are used to define a string.
3. Run the Program
IDLE: Press F5 or go to Run > Run Module.
Command Line/Terminal: Navigate to the file’s location and type:
python first_program.py
Output:
Hello, World!
Congratulations! You’ve just written and executed your first Python program.
Step 3: Understanding the Basics
Let’s break down the essentials of Python programming.
1. Comments
Comments are lines of text ignored by Python. They’re used to explain your code.
# This is a single-line comment
print("Hello, World!") # This prints a greeting
2. Variables
Variables store data for later use. Assign values using the =
operator.
# Storing data in variables
name = "Alice"
age = 25
print(f"My name is {name} and I am {age} years old.")
3. Input and Output
Use the input()
function to take input from the user and print()
to display output.
# Taking user input
name = input("What is your name? ")
print(f"Hello, {name}!")
Output:
What is your name? Alice
Hello, Alice!
4. Basic Arithmetic
Python supports basic arithmetic operations like addition, subtraction, multiplication, and division.
# Arithmetic operations
x = 10
y = 3
print(x + y) # Addition: 13
print(x - y) # Subtraction: 7
print(x * y) # Multiplication: 30
print(x / y) # Division: 3.333...
5. Conditional Statements
Control the flow of your program using if
, elif
, and else
.
# Checking conditions
age = int(input("Enter your age: "))
if age < 18:
print("You are a minor.")
elif age == 18:
print("Welcome to adulthood!")
else:
print("You are an adult.")
6. Loops
Python supports two types of loops: for
and while
.
# Loop through a range of numbers
for i in range(5):
print(i)
While Loop:
# Repeat until a condition is met
count = 0
while count < 5:
print(count)
count += 1
Step 4: Expanding Your Knowledge
Once you’ve mastered the basics, explore these topics to deepen your Python skills:
Data Structures: Learn about lists, dictionaries, and tuples.
Functions: Write reusable blocks of code using
def
.Modules: Use built-in or third-party modules to extend Python’s functionality.
File Handling: Learn to read and write files.
Example: A Simple Program
Let’s combine everything we’ve learned to write a simple calculator.
# Simple calculator program
num1 = float(input("Enter the first number: "))
num2 = float(input("Enter the second number: "))
print("Select operation:")
print("1. Add")
print("2. Subtract")
print("3. Multiply")
print("4. Divide")
choice = input("Enter your choice (1/2/3/4): ")
if choice == '1':
print(f"The result is: {num1 + num2}")
elif choice == '2':
print(f"The result is: {num1 - num2}")
elif choice == '3':
print(f"The result is: {num1 * num2}")
elif choice == '4':
if num2 != 0:
print(f"The result is: {num1 / num2}")
else:
print("Error: Division by zero is not allowed.")
else:
print("Invalid choice!")
Sample Run:
Enter the first number: 10
Enter the second number: 5
Select operation:
1. Add
2. Subtract
3. Multiply
4. Divide
Enter your choice (1/2/3/4): 1
The result is: 15.0
Conclusion
In just a few minutes, you’ve learned how to set up Python, write your first program, and understand the basics of variables, input/output, and logic. Python’s simplicity and power make it a great choice for beginners and professionals alike.
Practice what you’ve learned, experiment with different ideas, and take your first steps into the world of programming. With Python, the possibilities are endless!
Happy coding!