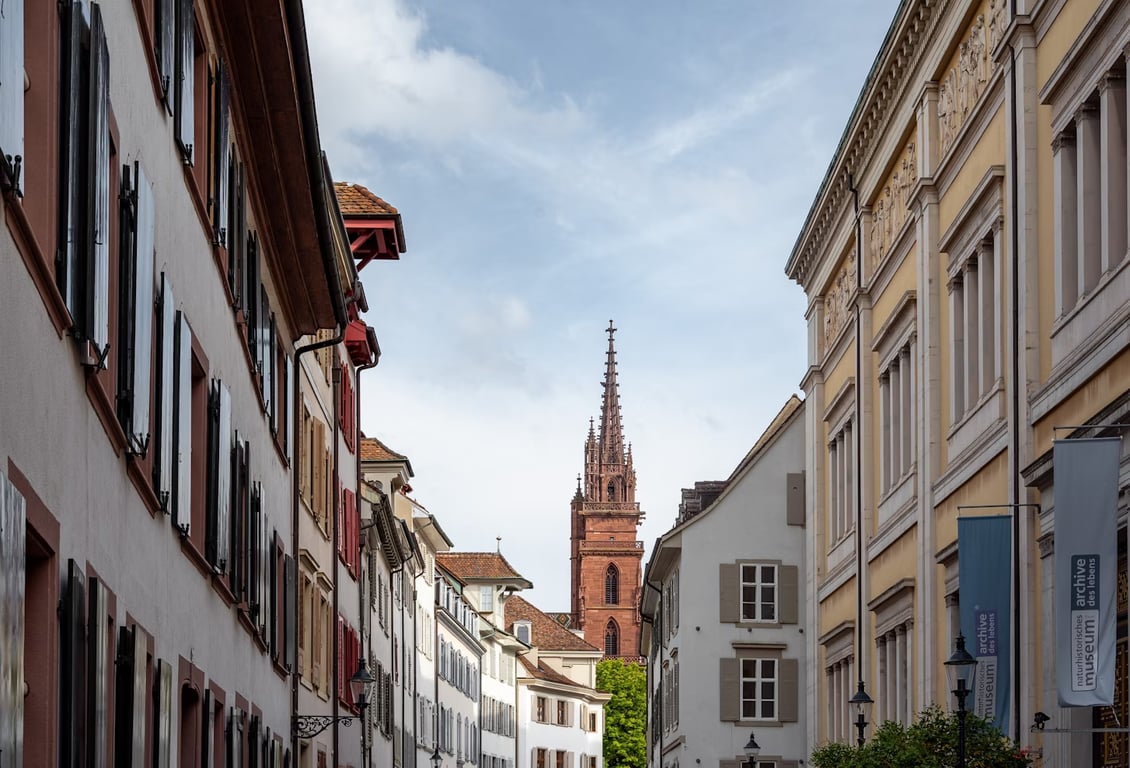
Photo by Anna Stampfli on Unsplash
Python's standard library is renowned for its simplicity and power, and the collections
module is a shining example. This module provides specialized container data types beyond the traditional list
, dict
, set
, and tuple
. These container types are optimized for specific use cases and help write more efficient and readable code.
In this guide, we will explore the key classes in Python's collections
module, their functionalities, and practical examples for each. By the end of this article, you’ll understand how to harness the full potential of the collections
module to simplify complex programming tasks.
Introduction to the collections
Module
The collections
module provides several high-performance, specialized container data types. These include:
namedtuple
: Factory function for creating tuple subclasses with named fields.deque
: A double-ended queue optimized for fast appends and pops.Counter
: A dictionary subclass for counting hashable objects.OrderedDict
: A dictionary that remembers the insertion order of keys.defaultdict
: A dictionary that provides default values for missing keys.ChainMap
: A class for managing multiple dictionaries as a single unit.UserDict
,UserList
,UserString
: Wrappers for customizing standard container types.
Each of these classes is designed for specific use cases. Let’s explore them one by one.
1. namedtuple
: Enhanced Tuples with Named Fields
The namedtuple
factory function creates tuple-like objects with named fields, making your code more self-documenting and readable.
Syntax:
from collections import namedtuple
Example:
from collections import namedtuple
# Define a namedtuple
Point = namedtuple('Point', ['x', 'y'])
# Create instances
p1 = Point(10, 20)
print(f"Point: {p1}, X: {p1.x}, Y: {p1.y}")
Output:
Point: Point(x=10, y=20), X: 10, Y: 20
Use Case:
Representing structured data like coordinates, database rows, or records.
2. deque
: Double-Ended Queues
deque
(pronounced "deck") is an optimized list-like container for fast operations at both ends. It’s ideal for implementing queues and stacks.
Syntax:
from collections import deque
Example:
from collections import deque
# Create a deque
dq = deque([1, 2, 3])
dq.append(4) # Add to the right
dq.appendleft(0) # Add to the left
print("Deque:", dq)
dq.pop() # Remove from the right
dq.popleft() # Remove from the left
print("Updated Deque:", dq)
Output:
Deque: deque([0, 1, 2, 3, 4])
Updated Deque: deque([1, 2, 3])
Use Case:
Implementing efficient FIFO/LIFO queues.
Sliding window algorithms.
3. Counter
: Counting Hashable Objects
Counter
is a dictionary subclass designed for counting the occurrences of items in an iterable.
Syntax:
from collections import Counter
Example:
from collections import Counter
# Count items in a list
fruits = ['apple', 'banana', 'apple', 'orange', 'banana', 'banana']
fruit_counter = Counter(fruits)
print(fruit_counter)
# Most common items
print("Most Common:", fruit_counter.most_common(2))
Output:
Counter({'banana': 3, 'apple': 2, 'orange': 1})
Most Common: [('banana', 3), ('apple', 2)]
Use Case:
Counting items in logs, survey responses, or datasets.
4. OrderedDict
: Maintaining Insertion Order
Before Python 3.7, dictionaries didn’t guarantee insertion order. OrderedDict
ensures that the order of key-value pairs is preserved.
Syntax:
from collections import OrderedDict
Example:
from collections import OrderedDict
# Create an OrderedDict
od = OrderedDict()
od['a'] = 1
od['b'] = 2
od['c'] = 3
print("OrderedDict:", od)
# Reverse the order
od.move_to_end('a')
print("After Moving 'a' to End:", od)
Output:
OrderedDict: OrderedDict([('a', 1), ('b', 2), ('c', 3)])
After Moving 'a' to End: OrderedDict([('b', 2), ('c', 3), ('a', 1)])
Use Case:
When order matters in a dictionary, such as in caching or configuration files.
5. defaultdict
: Dictionaries with Default Values
defaultdict
simplifies working with dictionaries that require default values for missing keys.
Syntax:
from collections import defaultdict
Example:
from collections import defaultdict
# Create a defaultdict with a default value of 0
dd = defaultdict(int)
dd['a'] += 1
dd['b'] += 2
print(dd)
Output:
defaultdict(<class 'int'>, {'a': 1, 'b': 2})
Use Case:
Grouping data, counting occurrences, or initializing complex structures.
6. ChainMap
: Merging Multiple Dictionaries
ChainMap
allows you to combine multiple dictionaries into a single, unified view.
Syntax:
from collections import ChainMap
Example:
from collections import ChainMap
# Combine dictionaries
dict1 = {'a': 1, 'b': 2}
dict2 = {'b': 3, 'c': 4}
chain = ChainMap(dict1, dict2)
print("ChainMap:", chain)
print("Value of 'b':", chain['b'])
Output:
ChainMap: ChainMap({'a': 1, 'b': 2}, {'b': 3, 'c': 4})
Value of 'b': 2
Use Case:
Overriding configurations by combining default and user-defined settings.
7. UserDict
, UserList
, and UserString
: Customizing Standard Containers
These classes are wrappers around Python's built-in containers, allowing you to extend or customize their behavior.
Example:
from collections import UserDict
class MyDict(UserDict):
def __setitem__(self, key, value):
print(f"Setting {key} to {value}")
super().__setitem__(key, value)
# Example Usage
d = MyDict()
d['a'] = 10
Output:
Setting a to 10
Use Case:
Extending or modifying the behavior of dictionaries, lists, or strings.
Advantages of the collections
Module
Efficiency: Specialized data types like
deque
andCounter
are optimized for specific tasks, improving performance.Readability: Classes like
namedtuple
anddefaultdict
make code more self-explanatory.Flexibility: Tools like
ChainMap
simplify managing complex configurations or data hierarchies.
Conclusion
Python’s collections
module is a treasure trove for developers, offering specialized container types to solve common problems efficiently. Whether you’re dealing with structured data, managing configurations, or counting items, the tools provided by this module can simplify your code and improve its performance.
By mastering these classes, you can write cleaner, more efficient Python programs while reducing the complexity of your code. So, explore the collections
module and see how it can transform the way you write Python!
Happy coding!