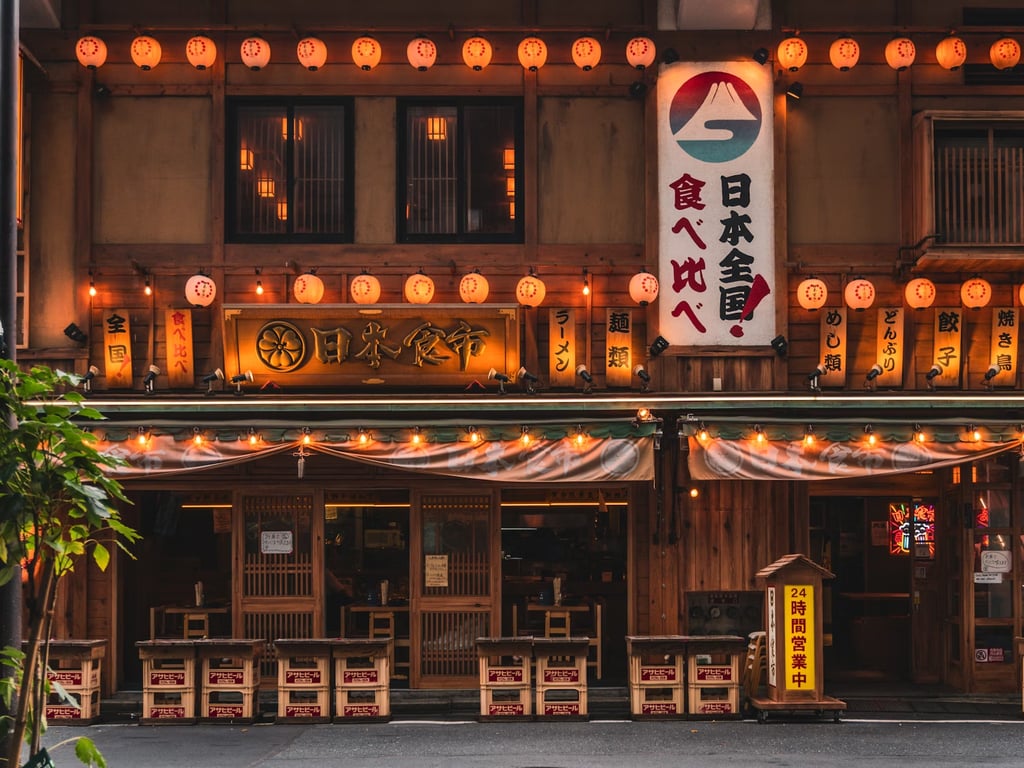
Photo by Kyle Hinkson on Unsplash
Python dictionaries are one of the most versatile and powerful data structures available. They enable you to store data in key-value pairs, making it easy to organize and access information. Whether you're a beginner or an experienced developer, understanding dictionaries is crucial for writing efficient and readable Python code.
In this blog, we’ll dive deep into Python dictionaries, their features, use cases, and real-world examples to help you master this essential data structure.
What Is a Dictionary in Python?
A Python dictionary is an unordered, mutable collection of key-value pairs. Each key is unique, and it maps to a corresponding value. Dictionaries are often used to store data that can be quickly retrieved using keys.
Key Features of Dictionaries:
Key-Value Pairs: Data is stored in pairs, where the key acts as a unique identifier.
Mutable: You can modify, add, or remove key-value pairs.
Unordered (Before Python 3.7): Keys are not stored in any specific order. From Python 3.7+, dictionaries maintain insertion order.
Dynamic: They can grow or shrink as needed.
Keys Must Be Immutable: Keys can be strings, numbers, or tuples but not lists or other mutable types.
Syntax of a Dictionary
# Creating a dictionary
person = {
"name": "Alice",
"age": 30,
"city": "New York"
}
Basic Operations with Dictionaries
1. Accessing Values
Use square brackets or the get()
method to retrieve values by their keys.
person = {"name": "Alice", "age": 30, "city": "New York"}
# Accessing values
print(person["name"]) # Output: Alice
print(person.get("age")) # Output: 30
2. Adding and Updating Key-Value Pairs
You can add a new key-value pair or update an existing one.
# Adding a new key-value pair
person["profession"] = "Engineer"
# Updating an existing value
person["age"] = 31
print(person)
# Output: {'name': 'Alice', 'age': 31, 'city': 'New York', 'profession': 'Engineer'}
3. Removing Elements
You can remove elements using pop()
, popitem()
, or del
.
# Using pop() to remove a key-value pair
person.pop("city")
# Using del to delete a key-value pair
del person["age"]
print(person)
# Output: {'name': 'Alice', 'profession': 'Engineer'}
4. Iterating Through a Dictionary
You can iterate over keys, values, or both using loops.
for key in person:
print(key, person[key]) # Output: name Alice, profession Engineer
Or, use the items()
method for key-value pairs:
for key, value in person.items():
print(f"{key}: {value}")
# Output:
# name: Alice
# profession: Engineer
5. Checking for Keys
Use the in
keyword to check if a key exists in the dictionary.
if "name" in person:
print("Name is a key.") # Output: Name is a key.
Real-World Examples of Python Dictionaries
1. Storing User Data
Dictionaries are ideal for storing user information.
user = {
"username": "john_doe",
"email": "john@example.com",
"age": 25,
"is_active": True
}
print(user["email"]) # Output: john@example.com
2. Word Frequency Counter
Dictionaries are commonly used to count occurrences of words in a text.
text = "apple banana apple orange banana apple"
words = text.split()
word_count = {}
for word in words:
word_count[word] = word_count.get(word, 0) + 1
print(word_count)
# Output: {'apple': 3, 'banana': 2, 'orange': 1}
3. Representing JSON Data
Dictionaries map naturally to JSON, which is widely used in web APIs.
import json
# JSON data
json_data = '{"name": "Alice", "age": 30, "city": "New York"}'
# Convert JSON to dictionary
data = json.loads(json_data)
print(data["city"]) # Output: New York
4. Nested Dictionaries
Dictionaries can store other dictionaries, allowing you to represent more complex data.
company = {
"name": "Tech Corp",
"employees": {
"john": {"age": 28, "role": "Developer"},
"mary": {"age": 34, "role": "Manager"}
}
}
print(company["employees"]["john"]["role"]) # Output: Developer
5. Building an Address Book
Create an address book where names are keys and contact details are values.
address_book = {
"Alice": {"phone": "123-456-7890", "email": "alice@example.com"},
"Bob": {"phone": "987-654-3210", "email": "bob@example.com"}
}
print(address_book["Alice"]["email"]) # Output: alice@example.com
6. Caching Data
Dictionaries can act as caches for storing and retrieving data quickly.
cache = {}
def factorial(n):
if n in cache:
return cache[n]
if n == 0:
return 1
result = n * factorial(n - 1)
cache[n] = result
return result
print(factorial(5)) # Output: 120
7. Mapping Students to Grades
Store student names and their grades.
grades = {
"Alice": "A",
"Bob": "B",
"Charlie": "A"
}
for student, grade in grades.items():
print(f"{student} got grade {grade}")
# Output:
# Alice got grade A
# Bob got grade B
# Charlie got grade A
Advanced Dictionary Techniques
1. Dictionary Comprehensions
Create dictionaries in a single line using comprehensions.
# Square of numbers as dictionary
squares = {x: x**2 for x in range(5)}
print(squares) # Output: {0: 0, 1: 1, 2: 4, 3: 9, 4: 16}
2. Default Dictionaries
Use collections.defaultdict
for dictionaries with default values.
from collections import defaultdict
word_count = defaultdict(int)
words = ["apple", "banana", "apple", "orange"]
for word in words:
word_count[word] += 1
print(word_count)
# Output: defaultdict(<class 'int'>, {'apple': 2, 'banana': 1, 'orange': 1})
3. Merging Dictionaries
Merge two dictionaries using the update()
method or the |
operator (Python 3.9+).
dict1 = {"a": 1, "b": 2}
dict2 = {"b": 3, "c": 4}
# Using update()
dict1.update(dict2)
print(dict1) # Output: {'a': 1, 'b': 3, 'c': 4}
# Using | operator
merged = dict1 | dict2
print(merged) # Output: {'a': 1, 'b': 3, 'c': 4}
Best Practices for Using Dictionaries
Choose Descriptive Keys: Use meaningful keys to make your code readable.
user = {"name": "Alice", "age": 30} # Clear and readable
Avoid Mutable Keys: Use immutable types (e.g., strings, numbers, or tuples) as keys.
Handle Missing Keys Gracefully: Use
get()
ordefaultdict
to avoidKeyError
.Use Nesting Wisely: Don’t over-nest dictionaries, as it can make your code hard to read.
Conclusion
Python dictionaries are a cornerstone of the language, offering unmatched flexibility and power. From simple key-value mappings to complex nested structures, dictionaries are indispensable in a wide range of programming tasks.
By mastering dictionaries, you’ll be able to write cleaner, more efficient Python code. Experiment with the examples and techniques provided in this blog to solidify your understanding and apply dictionaries to real-world scenarios.
Happy coding!