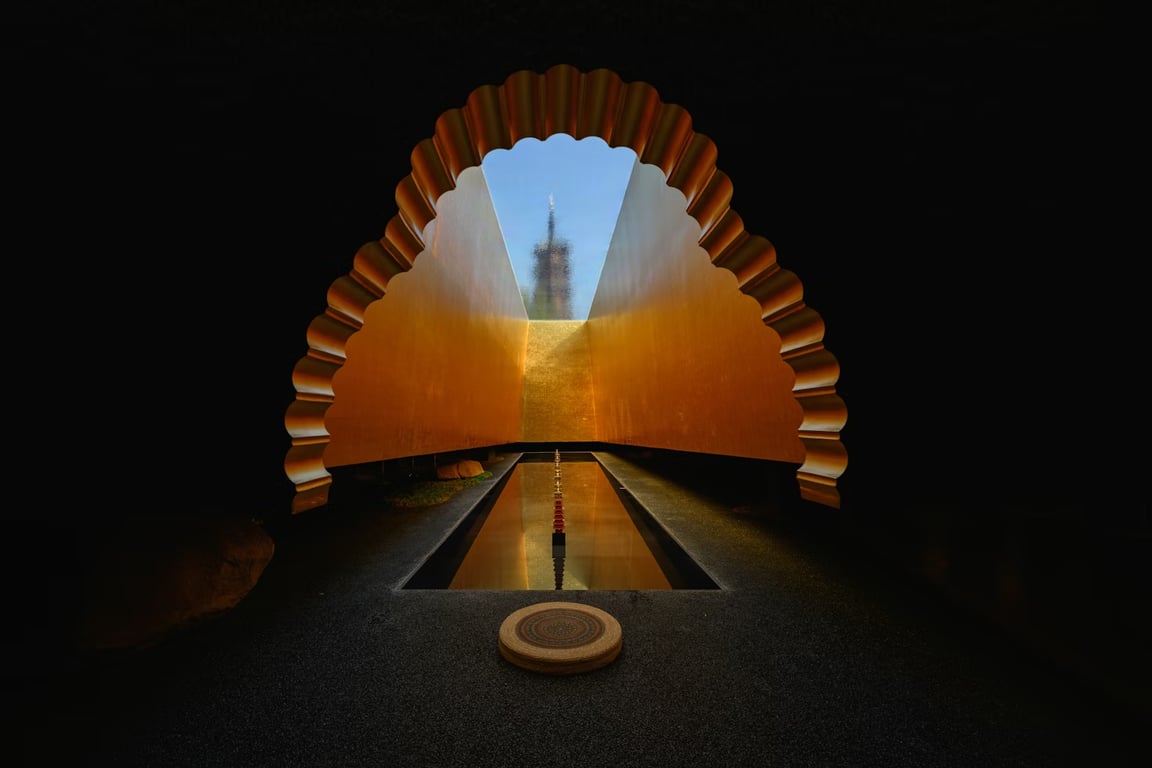
Photo by Declan Sun on Unsplash
When working with data structures in Python, you’ll often come across lists and tuples. Both are fundamental and widely used, but they serve different purposes. While lists are dynamic and versatile, tuples are immutable and efficient.
In this blog, we’ll explore the key differences between lists and tuples, when to use each, and practical examples to help you make the best choice in your Python programs.
What Are Lists and Tuples?
Lists
A list in Python is an ordered collection of items that are mutable, meaning you can add, remove, or modify elements after the list is created.
Characteristics of ListsMutable: You can change their content.
Dynamic: They can grow or shrink in size.
Heterogeneous: Can store elements of different data types.
# Creating a list
fruits = ["apple", "banana", "cherry"]
fruits.append("orange") # Adding an item
print(fruits) # Output: ['apple', 'banana', 'cherry', 'orange']
Tuples
A tuple is an ordered collection of items that are immutable, meaning their content cannot be changed after they are created.
Characteristics of TuplesImmutable: Cannot be modified.
Fixed Size: Once created, their size cannot change.
Heterogeneous: Like lists, tuples can store elements of different data types.
# Creating a tuple
coordinates = (10, 20, 30)
print(coordinates) # Output: (10, 20, 30)
Key Differences Between Lists and Tuples
List
Mutability : List is Mutable Data Structure
Syntax : List use Square brackets
[]
Performance : List is Slower (due to mutability overhead)
Use Cases : Dynamic data
Size : Can grow/shrink
Tuple
Mutability : List is Immutable Data Structure
Syntax : Tuple use Parentheses
()
Performance : List is Faster
Use Cases : Static or fixed data
Size : Fixed once created
When to Use Lists
1. When You Need to Modify Data
If you need to frequently add, remove, or change elements, lists are the ideal choice.
Example: Managing a To-Do Listto_do = ["buy groceries", "clean house"]
to_do.append("exercise") # Adding a new task
to_do[1] = "clean the car" # Modifying an existing task
print(to_do) # Output: ['buy groceries', 'clean the car', 'exercise']
2. When Data Size Is Dynamic
Lists are perfect for scenarios where the size of your data is not fixed and might grow or shrink during execution.
Example: Collecting User Inputuser_inputs = []
for i in range(3):
user_inputs.append(input("Enter a value: "))
print(user_inputs) # Output: List of user inputs
3. When You Need Advanced Methods
Lists come with many built-in methods like append()
, remove()
, sort()
, and reverse()
.
numbers = [5, 2, 8, 1]
numbers.sort() # Sorting in ascending order
print(numbers) # Output: [1, 2, 5, 8]
When to Use Tuples
1. When Data Should Not Change
Tuples are great for storing data that should remain constant throughout the program.
Example: Storing GPS Coordinatescoordinates = (37.7749, -122.4194) # Latitude, Longitude
print(coordinates) # Output: (37.7749, -122.4194)
2. When Performance Matters
Since tuples are immutable, they are faster and consume less memory than lists. Use them for large datasets where performance is critical.
Example: Large Dataset Processingdata_points = (1, 2, 3, 4, 5) # Immutable dataset
print(sum(data_points)) # Output: 15
3. As Dictionary Keys
Tuples can be used as dictionary keys, whereas lists cannot because they are mutable.
Example: Using Tuples as Keyslocations = {
(37.7749, -122.4194): "San Francisco",
(34.0522, -118.2437): "Los Angeles"
}
print(locations[(37.7749, -122.4194)]) # Output: San Francisco
Common Use Cases of Lists vs. Tuples
Lists in Real-World Applications
Dynamic Data Storage: For managing user-generated content or changing datasets.
Data Sorting and Searching: Built-in methods like
sort()
andindex()
make lists suitable for such tasks.Game Development: Storing player inventory or level data.
Tuples in Real-World Applications
Configuration Data: Use tuples for static settings or preferences.
Function Arguments: Returning multiple values from a function.
Immutable Records: Store unchangeable data like database records.
Examples Comparing Lists and Tuples
Example 1: Modifying Elements
Using a List:colors = ["red", "blue", "green"]
colors[1] = "yellow" # Modifying the second element
print(colors) # Output: ['red', 'yellow', 'green']
Using a Tuple:
colors = ("red", "blue", "green")
# colors[1] = "yellow" # Error: Tuples are immutable
Example 2: Memory Efficiency
List:import sys
list_data = [1, 2, 3, 4, 5]
print(sys.getsizeof(list_data)) # Memory usage of the list
Tuple:
import sys
tuple_data = (1, 2, 3, 4, 5)
print(sys.getsizeof(tuple_data)) # Memory usage of the tuple (less than list)
Example 3: Function Return Values
Tuples are often used to return multiple values from a function.
def calculate(a, b):
return a + b, a * b # Returning a tuple
result = calculate(5, 3)
print(result) # Output: (8, 15)
Pros and Cons of Lists and Tuples
Lists
Pros:Flexible and dynamic.
Rich set of built-in methods.
Slightly slower and more memory-intensive.
Less secure for constant data.
Tuples
Pros:Faster and more memory-efficient.
Ideal for fixed or constant data.
Cannot modify or resize after creation.
Conclusion
Both lists and tuples have their unique advantages and are suited to different use cases. Use lists when you need flexibility and dynamic data, and choose tuples when you need immutable, efficient, and secure data structures.
Understanding their differences and strengths allows you to write more optimized and efficient Python programs. With practice, you'll instinctively know when to use a list and when a tuple is the better choice.
Happy coding!