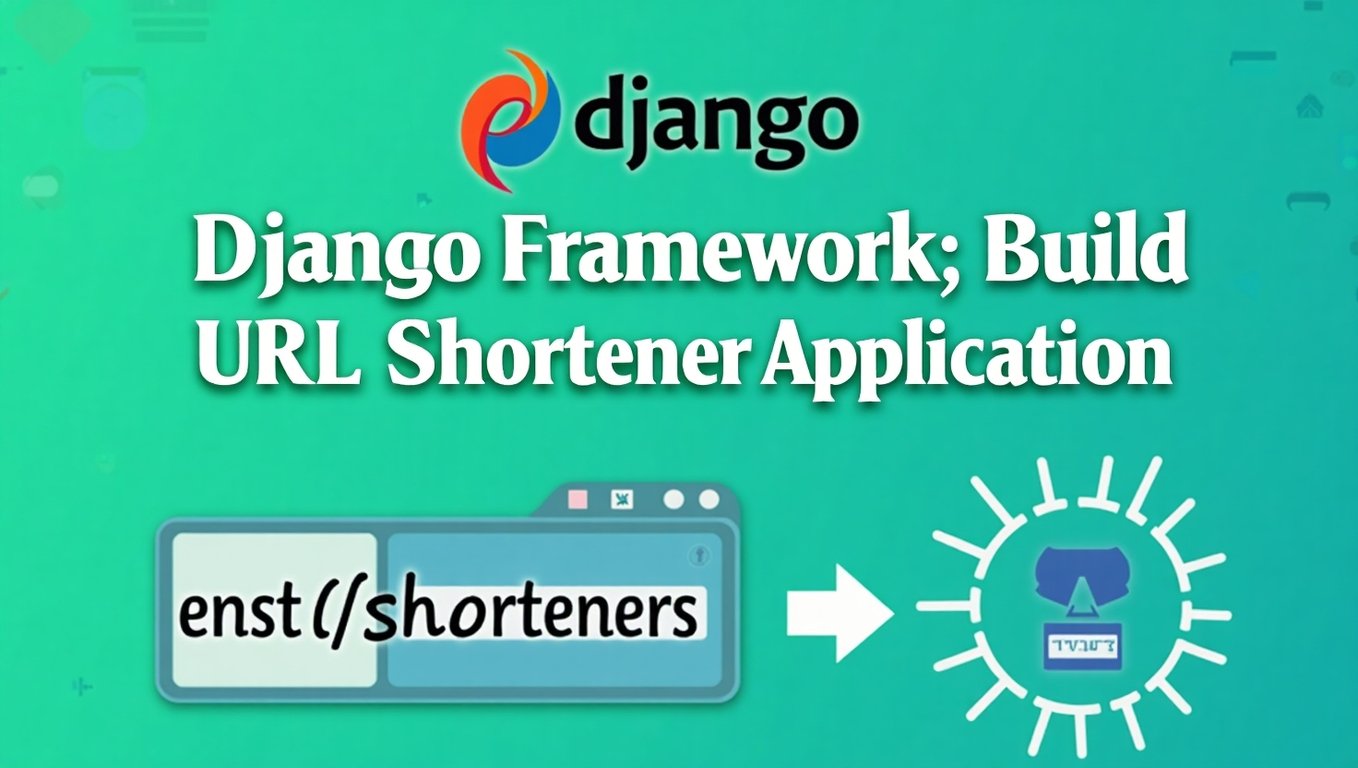
In this blog, we are going to build a simple URL Shortener application using django Framework and pyshortener package, that will going to shorten the long urls. By the end of this guide, you’ll be able to develop URL Shortener Django applications seamlessly.
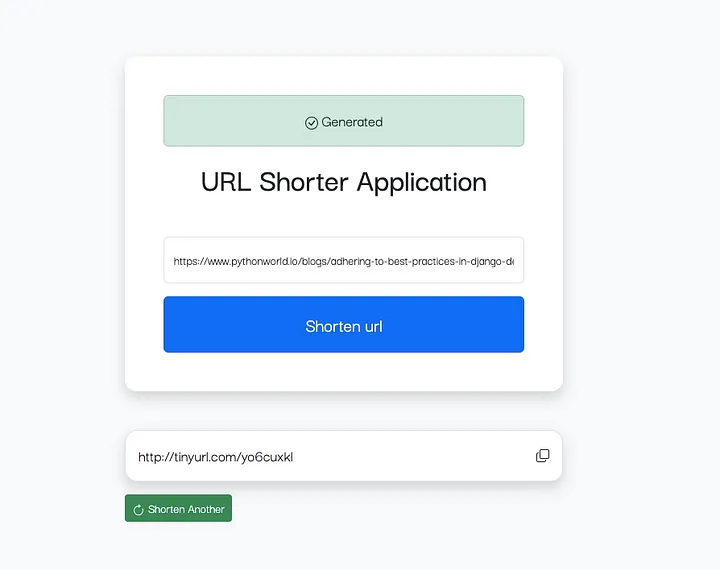
Install Dependencies
We need to install these two dependencies to start with this project :
Install django and pyshorteners by using this pip command
pip install django pyshorteners
It will install both dependencies in a single hit. Let’s move next to setup our django project.
Setup the Django Project
First, let’s create a simple django project by following these instructions :
Let’s create a django project i am giving a project name
urlshortener
.
django-admin startproject urlshortener
Next move inside to
urlshortener
project.
cd urlshortener
Now Let’s create a app i am giving a app name
main
.
python3 manage.py startapp main
Next, register our app under
INSTALLED_APPS
in settings.py file.
# urlshortener/settings.py
INSTALLED_APPS = [
...
'main.apps.MainConfig'
...
]
All set, our project is setup successfully. Cheers 🎉
Design the URL Shortener Application UI
Next we are going to build the UI for our URL Shortener application using django templates
.
We will create a templates/url-shortener.html
folder and file inside our main
app.
Next, we are going to write the UI code for our url shortener application in url-shortener.html
template file.
<!-- main/templates/url-shortener.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<title> URL Shortener <title/>
<!-- Boostrap CDN -->
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.2/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-T3c6CoIi6uLrA9TneNEoa7RxnatzjcDSCmG1MXxSR1GAsXEV/Dwwykc2MPK8M2HN" crossorigin="anonymous" />
<!-- Google fonts CDN -->
<link rel="preconnect" href="https://fonts.googleapis.com">
<rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<href="https://fonts.googleapis.com/css2?family=Darker+Grotesque:wght@300;400;500;600;700;800;900&display=swap" rel="stylesheet">
<!-- CSS Code -->
<style>
body{
font-family: 'Darker Grotesque', sans-serif;
}
</style>
</head>
<body class="bg-light">
<div class="container" style="width: 40%;margin-top: 5rem;">
<!-- Flash Message -->
<div class="p-5 text-center shadow bg-white" style="border-radius: 15px;">
{% if messages %}
{% for message in messages %}
<div class="alert alert-success text-center fs-5">
{{message}}
</div>
{% endfor %}
{% endif %}
</div>
<!-- Url Shortener Form -->
<h1>URL Shorter Application</h1>
<div class="mt-5">
<form action="" method="post">
{% csrf_token %}
<input type="url" name="url" value="{{url}}" required class="form-control py-3" placeholder="Enter long link here">
<div class="mt-3 d-grid">
<button class="btn btn-primary p-3 fs-4 text-white">Shorten url<button/>
</div>
</form>
</div>
<!-- Result Container -->
{% if messages %}
<div class="mt-5 bg-white border p-3 shadow" style="border-radius: 15px;">
<div class="d-flex align-items-center justify-content-between">
<span id="short-url" class="fs-5">{{short_url}}</span>
</div>
</div>
<div class="mt-3">
<a href="" class="btn btn-success btn-sm fs-6">Shorten Another</a>
</div>
{% endif %}
</div>
<!-- Javascript Code -->
<script>
const copy_url = ()=>{
const short_url = document.getElementById("short-url").innerText
navigator.clipboard.writeText(short_url);
}
</script>
</body>
</html>
Write the URL Shortener logic in view function
Next, we are going to create a view function, there we will implement the logic for url shortener.
# main/views.py
from django.shortcuts import render
import pyshorteners
from django.contrib import messages
def url_shortener(request):
try:
short_url = ""
url = ""
if request.method == "POST":
shortener = pyshorteners.Shortener()
url = request.POST.get("url")
short_url = shortener.tinyurl.short(url)
messages.success(request, "Generated")
return render(
request,"url-shortener.html", {"short_url": short_url, "url": url}
)
except:
returnrender(request, "url-shortener.html")
Next, just connect the url to this view
# main/urls.py
from .views import url_shortener
from django.urls import path
urlpatterns = [
path("", url_shortener, name="url-shortener"),
]
Run the server
Just hit this command to run the server and enjoy the application
python manage.py runserver
Conclusion
This is the simple url shortener application we have build in the article. You can even add more features to this url shortener application like generate QRCode for this url etc.
You can find the complete code of this project on my github account. If you want add something you can feel free to reach out to me.
Happy coding. :)