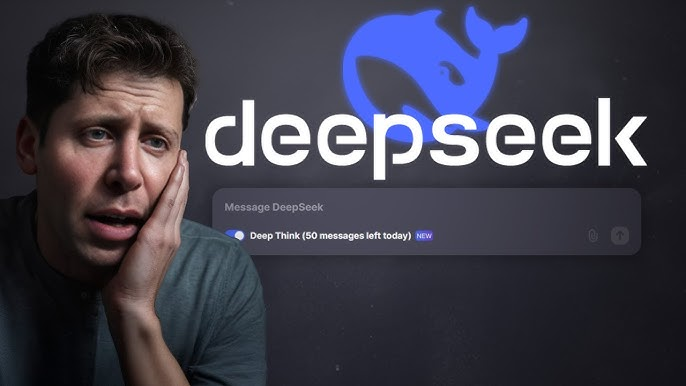
Artificial Intelligence (AI) is transforming web applications by enabling features like chatbots, text summarization, sentiment analysis, and personalized recommendations. Django, a high-level Python web framework, can easily integrate with AI-powered APIs such as OpenAI (ChatGPT, GPT-4) and DeepSeek to enhance application functionalities.
In this blog, we’ll explore how to:
✅ Integrate OpenAI & DeepSeek APIs into Django
✅ Build an AI-powered chatbot using OpenAI’s API
✅ Implement text summarization using DeepSeek API
✅ Handle API rate limits & errors
✅ Optimize Django’s performance with caching
By the end of this guide, you’ll be able to leverage AI models in your Django applications seamlessly. 🚀
Table of Contents
Introduction
Why Integrate AI Models with Django?
Setting Up OpenAI API in Django
Setting Up DeepSeek API in Django
Implementing an AI Chatbot using OpenAI
Implementing Text Summarization using DeepSeek
Handling API Rate Limits and Errors
Optimizing Performance with Caching
Deploying AI-Powered Django Applications
Conclusion
1. Introduction
Django, known for its clean design and scalability, can easily integrate AI models using RESTful APIs. Instead of training machine learning models from scratch, developers can use OpenAI and DeepSeek APIs to perform:
✔ Chatbot interactions (e.g., customer support, FAQs)
✔ Text summarization (e.g., summarizing blog articles)
✔ Sentiment analysis (e.g., detecting user emotions in feedback)
✔ Personalized recommendations (e.g., AI-powered product suggestions)
With simple API calls, we can enhance Django applications with AI capabilities.
2. Why Integrate AI Models with Django?
Using AI models in Django applications brings multiple benefits:
✅ No Need to Train AI Models
Pre-trained AI models like GPT-4 and DeepSeek allow developers to integrate cutting-edge AI without requiring ML expertise.
✅ Scalability & Performance
Cloud-based AI models handle large-scale requests efficiently, making them ideal for Django’s scalable architecture.
✅ Enhanced User Experience
AI-powered chatbots and content generation tools improve user engagement by automating responses and personalizing content.
3. Setting Up OpenAI API in Django
Step 1: Get an OpenAI API Key
Create an account at OpenAI.
Get your API Key from the dashboard.
Step 2: Install OpenAI Python SDK
pip install openai
Step 3: Configure OpenAI API in Django
Add your OpenAI API key in settings.py:
import os
OPENAI_API_KEY = os.getenv("OPENAI_API_KEY", "your-openai-api-key-here")
Step 4: Using OpenAI API in Django
Example: Generating AI responses in Django
import openai
from django.conf import settings
def generate_response(prompt):
response = openai.ChatCompletion.create(
model="gpt-4",
messages=[{"role": "user", "content": prompt}],
api_key=settings.OPENAI_API_KEY
)
return response["choices"][0]["message"]["content"]
Now, you can generate AI-powered responses dynamically in your Django app.
4. Setting Up DeepSeek API in Django
Step 1: Get a DeepSeek API Key
Sign up at DeepSeek and obtain an API key.
Step 2: Install Required Libraries
pip install requests
Step 3: Configure DeepSeek API in Django
Add the API key in settings.py:
import os
DEEPSEEK_API_KEY = os.getenv("DEEPSEEK_API_KEY", "your-deepseek-api-key-here")
Step 4: Using DeepSeek API in Django
Example: Fetching AI-generated text from DeepSeek
import requests
from django.conf import settings
def fetch_deepseek_response(prompt):
url = "https://api.deepseek.com/v1/generate"
headers = {"Authorization": f"Bearer {settings.DEEPSEEK_API_KEY}"}
payload = {"prompt": prompt, "max_tokens": 100}
response = requests.post(url, headers=headers, json=payload)
return response.json()["text"]
5. Implementing an AI Chatbot using OpenAI
Step 1: Create a Django View for Chatbot
from django.shortcuts import render
from django.http import JsonResponse
def chatbot(request):
if request.method == "POST":
user_message = request.POST.get("message")
ai_response = generate_response(user_message)
return JsonResponse({"response": ai_response})
return render(request, "chat.html")
Step 2: Create a Simple Chat UI
<form id="chat-form">
<input type="text" id="user-input" placeholder="Ask something...">
<button type="submit">Send</button>
</form>
<div id="chat-output"></div>
<script>
document.getElementById("chat-form").onsubmit = function(event) {
event.preventDefault();
let userInput = document.getElementById("user-input").value;
fetch("/chatbot/", {
method: "POST",
body: new URLSearchParams({message: userInput})
})
.then(response => response.json())
.then(data => {
document.getElementById("chat-output").innerHTML += `<p><strong>Bot:</strong> ${data.response}</p>`;
});
};
</script>
Now, your Django chatbot can respond to user queries using OpenAI’s API!
6. Implementing Text Summarization using DeepSeek
Step 1: Create a Django View for Summarization
from django.shortcuts import render
from django.http import JsonResponse
def summarize_text(request):
if request.method == "POST":
text = request.POST.get("text")
summary = fetch_deepseek_response(f"Summarize this: {text}")
return JsonResponse({"summary": summary})
return render(request, "summarize.html")
Step 2: Create a Simple UI for Summarization
<form id="summarize-form">
<textarea id="input-text" placeholder="Enter text to summarize..."></textarea>
<button type="submit">Summarize</button>
</form>
<div id="summary-output"></div>
Now, users can summarize text using DeepSeek’s AI API.
7. Handling API Rate Limits and Errors
Both OpenAI & DeepSeek APIs have rate limits. To handle them gracefully:
import time
def fetch_with_retry(api_call, retries=3, delay=2):
for attempt in range(retries):
try:
return api_call()
except requests.exceptions.RequestException:
time.sleep(delay)
return "Error: API request failed."
8. Optimizing Performance with Caching
Cache responses from AI APIs to reduce API costs:
from django.core.cache import cache
def get_cached_response(prompt):
cached_response = cache.get(prompt)
if cached_response:
return cached_response
response = generate_response(prompt)
cache.set(prompt, response, timeout=300) # Cache for 5 minutes
return response
9. Deploying AI-Powered Django Applications
Use Celery to run API requests asynchronously and Docker for deploying AI-powered Django apps efficiently.
10. Conclusion
✅ OpenAI & DeepSeek APIs enable AI features in Django
✅ Built chatbot & summarization examples
✅ Optimized performance with caching & rate limits
With these integrations, you can build powerful AI applications using Django! 🚀
Happy coding!