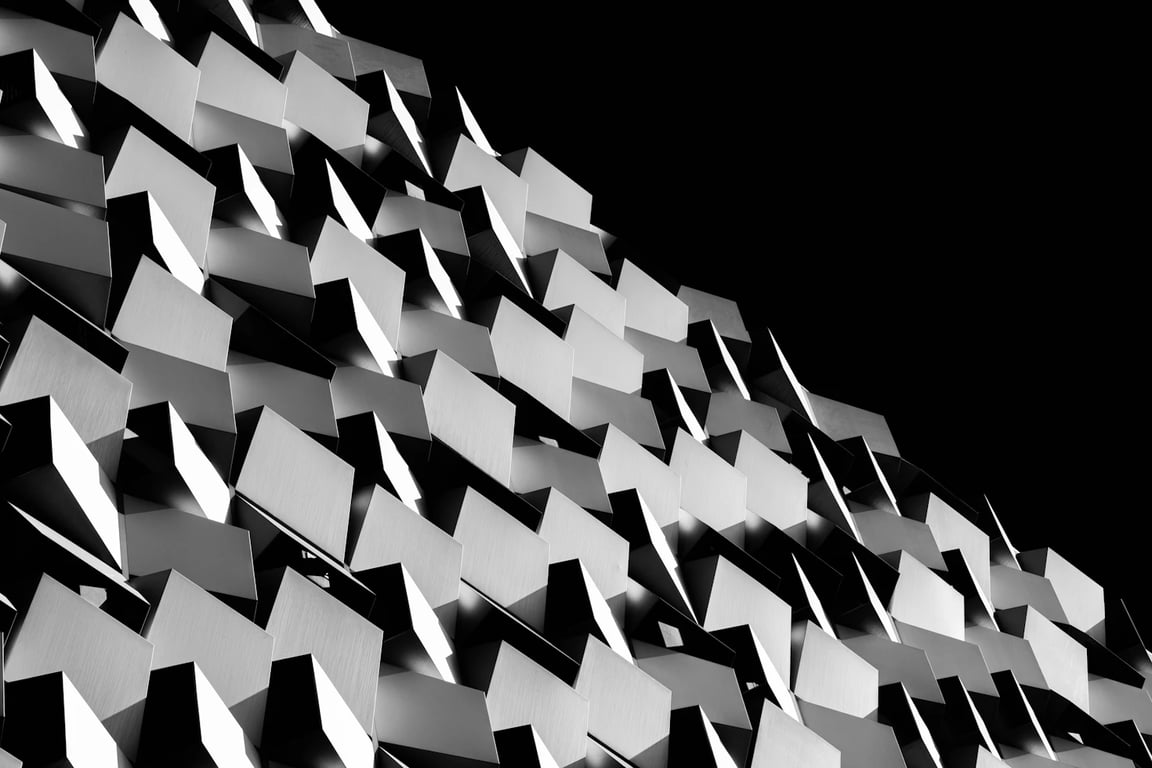
Photo by Mike Hindle on Unsplash
As web applications grow in complexity and traffic, traditional monolithic architectures often struggle to keep up with scalability and maintainability requirements. Django, a popular web framework, is typically used in a monolithic fashion. However, leveraging microservices architecture can significantly enhance a Django application’s scalability, fault tolerance, and development speed.
In this guide, we’ll explore how to build scalable Django applications using a microservices architecture, breaking down the advantages, challenges, and best practices with examples.
Table of Contents
What is Microservices Architecture?
Why Use Microservices in Django?
Setting Up a Microservices Architecture with Django
Step 1: Breaking Down the Application
Step 2: Creating Independent Django Microservices
Step 3: Communication Between Microservices
Step 4: Deploying Django Microservices with Docker
Conclusion
What is Microservices Architecture?
Microservices architecture is a design pattern in which an application is composed of small, independent services, each responsible for a specific functionality. These services communicate through APIs (often RESTful or gRPC) and are independently deployable.
Key Characteristics of Microservices:
Decentralized Development – Each service is developed, deployed, and maintained independently.
Scalability – Services can be scaled individually based on demand.
Fault Isolation – Failures in one service do not necessarily bring down the entire system.
Technology Agnostic – Different services can use different technologies.
Why Use Microservices in Django?
Django is often associated with monolithic applications, but it can be adapted for microservices with the right approach. Here’s why you might consider microservices for your Django application:
Handling High Traffic: Microservices allow independent scaling of specific services.
Better Maintainability: Teams can work on different services without affecting others.
Improved Performance: Services can be optimized individually for performance.
Easier Deployment & Updates: Updates can be pushed to specific services without downtime.
Setting Up a Microservices Architecture with Django
Step 1: Breaking Down the Application
Before implementing microservices, break your Django monolith into different independent services. For example, a typical e-commerce application might have:
User Service: Handles authentication and user profiles
Order Service: Manages orders and payments
Product Service: Manages product listings and inventory
Notification Service: Handles email and SMS notifications
Each service will run as an independent Django project, communicating with other services via REST APIs or message queues like RabbitMQ.
Step 2: Creating Independent Django Microservices
1. User Service (Authentication Microservice)
Initialize a Django project for authentication:
mkdir user_service && cd user_service
django-admin startproject user_service .
Create an API for user authentication using Django REST Framework (DRF):
# user_service/users/views.py
from rest_framework.decorators import api_view
from rest_framework.response import Response
from django.contrib.auth.models import User
@api_view(['POST'])
def register_user(request):
username = request.data.get('username')
password = request.data.get('password')
user = User.objects.create_user(username=username, password=password)
return Response({'message': 'User created successfully'})
Expose it via urls.py
:
from django.urls import path
from .views import register_user
urlpatterns = [
path('register/', register_user, name='register_user'),
]
Start the service:
python manage.py runserver 8001
This service will now handle authentication at http://localhost:8001/register/
.
2. Order Service (Orders Microservice)
Set up a new Django project for handling orders:
mkdir order_service && cd order_service
django-admin startproject order_service .
Define an Order model in models.py
:
from django.db import models
class Order(models.Model):
user_id = models.IntegerField()
product_id = models.IntegerField()
quantity = models.IntegerField()
status = models.CharField(max_length=20, default='Pending')
Create an API to place orders:
# order_service/orders/views.py
from rest_framework.decorators import api_view
from rest_framework.response import Response
from .models import Order
@api_view(['POST'])
def create_order(request):
user_id = request.data.get('user_id')
product_id = request.data.get('product_id')
quantity = request.data.get('quantity')
order = Order.objects.create(user_id=user_id, product_id=product_id, quantity=quantity)
return Response({'message': 'Order placed successfully', 'order_id': order.id})
Expose it via urls.py
:
from django.urls import path
from .views import create_order
urlpatterns = [
path('create/', create_order, name='create_order'),
]
Start this service on a different port:
python manage.py runserver 8002
The API will be accessible at http://localhost:8002/create/
.
Step 3: Communication Between Microservices
Django microservices can communicate via REST APIs or Message Queues (RabbitMQ, Kafka, etc.).
Calling the User Service from Order Service:Modify the create_order
function to validate the user before placing an order:
import requests
@api_view(['POST'])
def create_order(request):
user_id = request.data.get('user_id')
response = requests.get(f'http://localhost:8001/users/{user_id}/')
if response.status_code != 200:
return Response({'error': 'User not found'}, status=400)
product_id = request.data.get('product_id')
quantity = request.data.get('quantity')
order = Order.objects.create(user_id=user_id, product_id=product_id, quantity=quantity)
return Response({'message': 'Order placed successfully', 'order_id': order.id})
This ensures that only valid users can place orders.
Step 4: Deploying Django Microservices with Docker
Create a Dockerfile
for each service:
FROM python:3.9
WORKDIR /app
COPY requirements.txt .
RUN pip install -r requirements.txt
COPY . .
CMD ["python", "manage.py", "runserver", "0.0.0.0:8000"]
Use Docker Compose to manage multiple services:
version: '3'
services:
user_service:
build: ./user_service
ports:
- "8001:8001"
order_service:
build: ./order_service
ports:
- "8002:8002"
Run the services:
docker-compose up -d
Conclusion
Using microservices in Django enhances scalability, modularity, and maintainability. By breaking a monolithic Django application into separate microservices, you gain benefits like independent deployment, scalability, and fault tolerance. However, it also introduces challenges such as managing inter-service communication and deployments.
Start small by identifying modules that can be separated and incrementally migrate to a microservices architecture.
Happy coding! 🚀