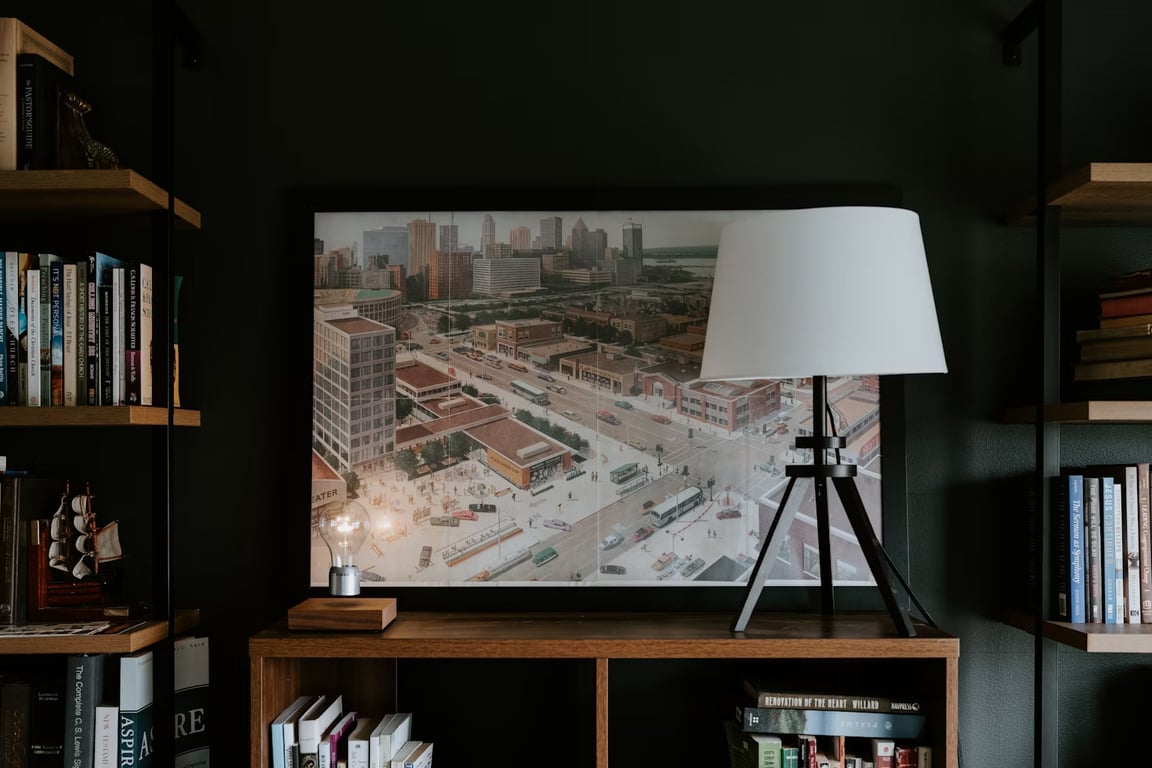
Photo by Kelly Sikkema on Unsplash
Control flow is the backbone of any programming language, dictating the sequence in which code executes. Python, known for its simplicity and readability, offers intuitive ways to manage control flow with constructs like if, for, and while statements. These fundamental tools allow developers to write programs that make decisions, iterate over data, and perform repetitive tasks effectively.
In this beginner-friendly guide, we will dive deep into these constructs, breaking down their syntax and usage with real-world examples to ensure clarity and ease of understanding.
Understanding Control Flow in Python
Control flow determines how your program makes decisions and executes instructions based on conditions or loops. Python's if, for, and while statements are crucial for implementing:
Conditional Logic (if statements): Decide which block of code to execute based on specific conditions.
Loops (for and while statements): Repeat a set of instructions until a condition is met.
The if Statement: Making Decisions
The if statement is used to execute a block of code only if a specified condition is true. It acts as the foundation of decision-making in Python.
Syntax:
if condition:
# Code to execute if the condition is true
elif another_condition:
# Code to execute if the previous condition is false but this one is true
else:
# Code to execute if all conditions are false
Example 1: Basic if Statement
age = 18
if age >= 18:
print("You are eligible to vote.")
Example 2: Using if-elif-else
score = 75
if score >= 90:
print("Grade: A")
elif score >= 75:
print("Grade: B")
else:
print("Grade: C")
Real-World Use Case: Traffic Light System
light = "red"
if light == "green":
print("Go!")
elif light == "yellow":
print("Slow down.")
else:
print("Stop!")
Key Points to Remember:
Indentation is mandatory in Python to define the block of code.
Conditions can use comparison (
==
,!=
,<
,>
), logical (and
,or
,not
), and membership operators (in
,not in
).
The for Loop: Iterating Over Sequences
The for loop is used to iterate over a sequence (like a list, tuple, string, or range). It repeats the block of code for each item in the sequence.
Syntax:
for variable in sequence:
# Code to execute for each item in the sequence
Example 1: Iterating Over a List
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
Example 2: Using range()
The range()
function generates a sequence of numbers.
number = 5
for i in range(1, 11):
print(f"{number} x {i} = {number * i}")
Real-World Use Case: Displaying Multiplication Table
number = 5
for i in range(1, 11):
print(f"{number} x {i} = {number * i}")
Nested for Loops
You can nest one loop inside another to handle multi-dimensional data.
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
for row in matrix:
for value in row:
print(value, end=" ")
print()
Key Points to Remember:
The
break
statement exits the loop prematurely.The
continue
statement skips the current iteration and moves to the next.
The while Loop: Looping With Conditions
The while loop executes a block of code as long as the specified condition is true. It's commonly used when the number of iterations is not known beforehand.
Syntax:
while condition:
# Code to execute while the condition is true
Example 1: Basic while Loop
count = 1
while count <= 5:
print(count)
count += 1
Example 2: Using break in while
number = 0
while True:
number += 1
if number == 5:
print("Loop exited at number 5")
break
Real-World Use Case: Password Validation
correct_password = "python123"
attempt = ""
while attempt != correct_password:
attempt = input("Enter the password: ")
if attempt == correct_password:
print("Access Granted!")
else:
print("Incorrect password, try again.")
Comparing for and while Loops
for Loop
Use Case : Iterates over a sequence (list, range)
When to Use : Known number of iterations
Examples : Traversing a list, printing numbers
while Loop
Use Case : Executes until a condition is false
When to Use : Unknown number of iterations
Examples : Validating input, waiting for events
Combining if, for, and while Statements
These constructs can be combined to create more complex logic in your programs.
Example: Number Guessing Game
import random
secret_number = random.randint(1, 10)
guess = 0
while guess != secret_number:
guess = int(input("Guess a number between 1 and 10: "))
if guess < secret_number:
print("Too low!")
elif guess > secret_number:
print("Too high!")
else:
print("Congratulations! You guessed it!")
Common Mistakes and How to Avoid Them
1. Forgetting to Update Loop Conditions
Infinite loops occur when the condition in a while
loop never becomes false.
# This will run indefinitely
count = 1
while count < 5:
print(count)
# Add count += 1 to fix this
2. Misusing Indentation
Python relies on indentation for defining blocks of code. Misaligned code can cause errors or unexpected behavior.
if True:
print("This will throw an IndentationError.") # Incorrect indentation
3. Using for
Instead of while
(and Vice Versa)
Choose the right loop based on the problem. Use for
when iterating over sequences and while
when conditions are dynamic.
Real-World Application of if, for, and while Statements
Example: Analyzing Shopping Cart Data
cart = [
{"item": "Laptop", "price": 800},
{"item": "Headphones", "price": 50},
{"item": "Mouse", "price": 20},
]
# Calculate total price and list expensive items
total_price = 0
expensive_items = []
for product in cart:
total_price += product["price"]
if product["price"] > 100:
expensive_items.append(product["item"])
print(f"Total Price: ${total_price}")
print(f"Expensive Items: {', '.join(expensive_items)}")
Conclusion
Mastering if, for, and while statements in Python is essential for building dynamic and robust applications. These constructs form the foundation of control flow, enabling your programs to make decisions and repeat tasks efficiently.
By understanding their syntax, use cases, and best practices, you can start writing Python code that is both effective and elegant. Practice combining these statements in real-world scenarios to deepen your understanding and enhance your programming skills.
Happy coding!