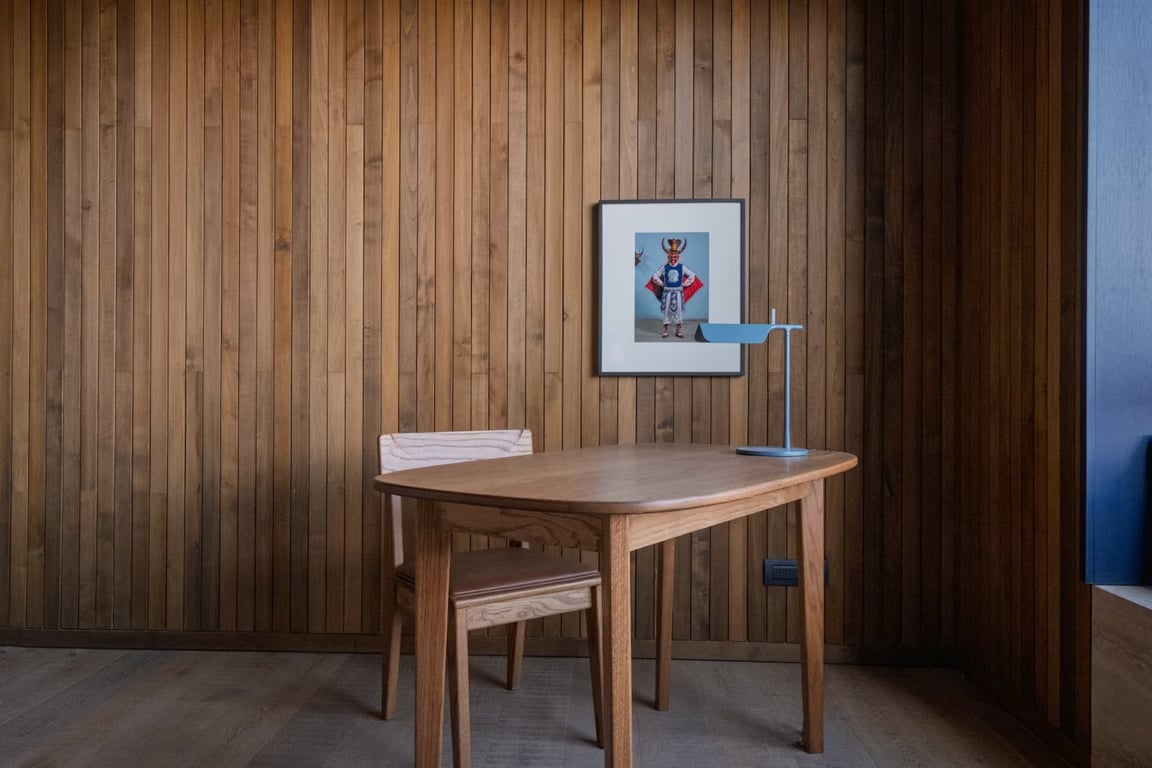
Photo by Diego Marín on Unsplash
Handling dates and times is a common task in programming, especially when dealing with applications related to logging, scheduling, and even financial systems. Python’s datetime
module offers an elegant and easy-to-use way to manage and manipulate date and time objects. Whether you’re calculating the difference between two dates, converting time formats, or working with time zones, Python’s datetime
module provides the necessary tools to help you.
In this blog, we’ll dive into the various functionalities provided by the datetime
module. We’ll explore how to handle dates, times, and both, including practical examples for each. By the end of this guide, you’ll be comfortable using the datetime
module to handle a variety of time-based operations in your Python programs.
What is the datetime Module?
The datetime
module is part of Python’s standard library, which means you don’t need to install any third-party libraries to use it. It supplies classes for working with dates, times, and even time intervals. The most commonly used classes within the datetime
module are:
datetime.date
- Used for manipulating dates (year, month, day).datetime.time
- Deals with time (hour, minute, second, microsecond).datetime.datetime
- Combines both date and time (year, month, day, hour, minute, second).datetime.timedelta
- Used for representing time intervals or differences between dates/times.datetime.tzinfo
- Base class for dealing with time zone information.
Getting Started: Importing datetime
To use the datetime
module, you first need to import it. You can import the entire module or just the specific classes you need.
import datetime
You can also import specific classes like date
, time
, or datetime
directly to avoid typing datetime.datetime
each time:
from datetime import datetime, date, time, timedelta
Working with Date Objects
The date
class in the datetime
module represents a date without time. You can easily create a date object using the date()
constructor, which requires the year, month, and day as arguments.
Creating a Date Object
import datetime
# Creating a date object
my_date = datetime.date(2025, 1, 13)
print(my_date) # Output: 2025-01-13
Getting the Current Date
To get the current date, you can use the today()
method, which returns the current local date.
current_date = datetime.date.today()
print("Today's Date:", current_date)
Accessing Date Attributes
Once you have a date object, you can access its individual components like year, month, and day.
my_date = datetime.date(2025, 1, 13)
print("Year:", my_date.year)
print("Month:", my_date.month)
print("Day:", my_date.day)
Working with Time Objects
The time
class in the datetime
module represents a time without any reference to a date. It is useful when you need to manipulate time alone.
Creating a Time Object
import datetime
# Creating a time object
my_time = datetime.time(14, 30, 15)
print(my_time) # Output: 14:30:15
Accessing Time Attributes
You can access hours, minutes, seconds, and microseconds of a time object.
my_time = datetime.time(14, 30, 15)
print("Hour:", my_time.hour)
print("Minute:", my_time.minute)
print("Second:", my_time.second)
Working with Datetime Objects
The datetime
class combines both date and time components. This is one of the most used classes in the datetime
module, and it’s perfect when you need both the date and time information.
Creating a Datetime Object
You can create a datetime
object by passing the year, month, day, hour, minute, second, and microsecond.
import datetime
# Creating a datetime object
my_datetime = datetime.datetime(2025, 1, 13, 14, 30, 15)
print(my_datetime) # Output: 2025-01-13 14:30:15
Getting the Current Date and Time
To get the current local date and time, use the now()
method.
current_datetime = datetime.datetime.now()
print("Current Datetime:", current_datetime)
Accessing Datetime Attributes
Just like with date and time objects, you can extract individual components from a datetime
object.
my_datetime = datetime.datetime(2025, 1, 13, 14, 30, 15)
print("Year:", my_datetime.year)
print("Month:", my_datetime.month)
print("Day:", my_datetime.day)
print("Hour:", my_datetime.hour)
print("Minute:", my_datetime.minute)
print("Second:", my_datetime.second)
Formatting Dates and Times
Formatting dates and times is crucial when you want to display them in a human-readable format or in a specific structure. The strftime()
method is used for formatting datetime
objects as strings.
Formatting Datetime Objects
current_datetime = datetime.datetime.now()
# Formatting the datetime object
formatted_datetime = current_datetime.strftime("%Y-%m-%d %H:%M:%S")
print("Formatted Datetime:", formatted_datetime)
Common Format Codes
Here are some commonly used format codes in strftime()
:
%Y
: Year with century (e.g., 2025)%m
: Month as a zero-padded decimal number (e.g., 01)%d
: Day of the month as a zero-padded decimal number (e.g., 13)%H
: Hour (24-hour clock) as a zero-padded decimal number (e.g., 14)%M
: Minute as a zero-padded decimal number (e.g., 30)%S
: Second as a zero-padded decimal number (e.g., 15)
Parsing Dates and Times
To convert a string representation of a date or time back into a datetime
object, you can use the strptime()
method.
date_string = "2025-01-13 14:30:15"
parsed_datetime = datetime.datetime.strptime(date_string, "%Y-%m-%d %H:%M:%S")
print("Parsed Datetime:", parsed_datetime)
Working with Timedelta Objects
The timedelta
class represents the difference between two datetime
objects. This is useful when you need to add or subtract time.
Creating a Timedelta Object
import datetime
# Creating a timedelta object representing 10 days
delta = datetime.timedelta(days=10)
# Getting the current date
current_date = datetime.date.today()
# Adding the timedelta to the current date
new_date = current_date + delta
print("New Date:", new_date)
Subtracting Dates
You can also subtract one datetime
or date
object from another, which results in a timedelta
object.
date1 = datetime.date(2025, 1, 13)
date2 = datetime.date(2025, 1, 1)
delta = date1 - date2
print("Difference in Days:", delta.days)
Time Zones and Timezone Awareness
Python’s datetime
module also provides support for time zones. You can make a datetime
object timezone-aware by associating it with a specific time zone using the pytz
library.
Timezone-Aware Datetime
import pytz
# Creating a timezone-aware datetime object
timezone = pytz.timezone("US/Eastern")
eastern_time = datetime.datetime.now(timezone)
print("Eastern Time:", eastern_time)
Conclusion
The datetime
module is a powerful tool for manipulating dates and times in Python. With its ability to handle everything from basic date manipulation to time zone support, it’s an essential library for any Python developer. By understanding how to create, format, and manipulate date
, time
, and datetime
objects, you can handle a wide variety of time-based tasks in your programs.
Whether you need to calculate time differences, convert formats, or work with time zones, the datetime
module provides the flexibility and functionality you need. As you practice with these examples and expand your knowledge, you’ll find that working with dates and times in Python becomes second nature.
Happy coding!