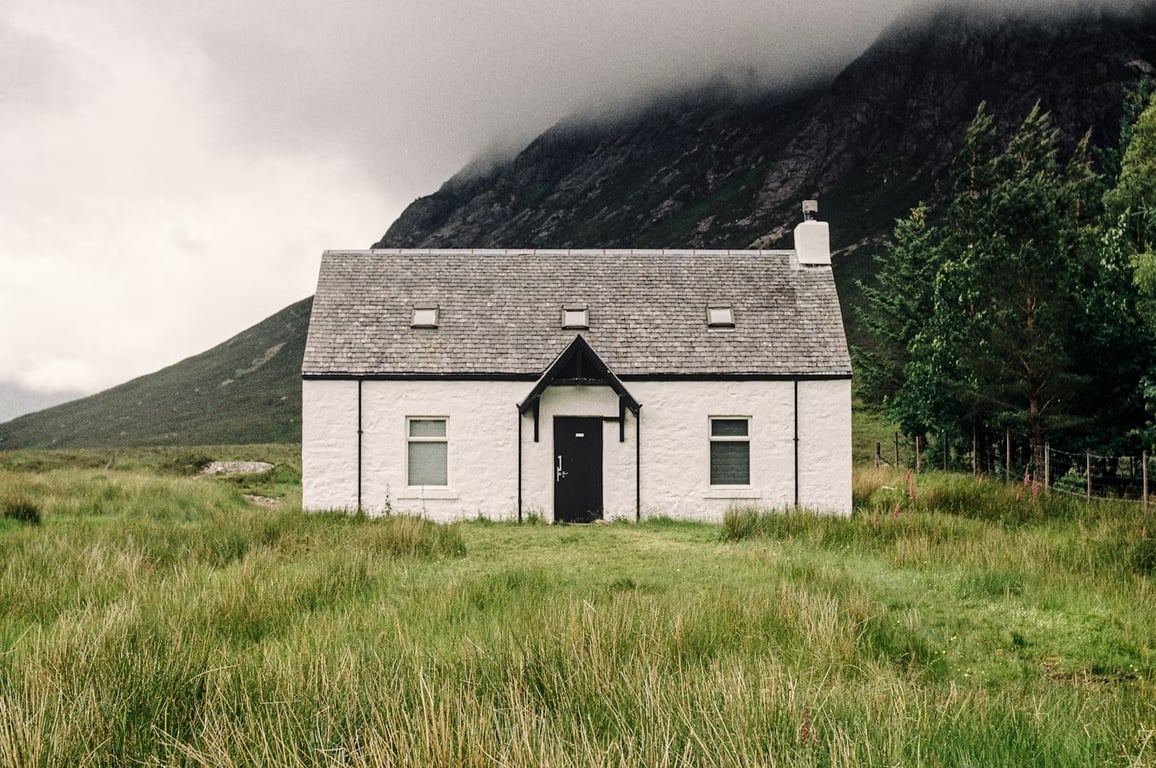
Photo by Alex Harwood on Unsplash
In today's interconnected world, APIs (Application Programming Interfaces) play a crucial role in enabling communication between applications. APIs allow developers to access data and functionality from other services, such as weather updates, social media feeds, or payment gateways. Python’s requests
library is one of the most popular tools for interacting with APIs, offering a user-friendly way to send HTTP requests and handle responses.
This guide provides an in-depth exploration of working with APIs using Python’s requests
library. Whether you're a beginner or an experienced developer, this blog will help you master the essentials.
What is an API?
An API is a set of rules and protocols that allow different software applications to communicate with each other. APIs can:
Enable applications to access remote data.
Allow services to expose specific functionality to developers.
Be categorized as REST (Representational State Transfer) or SOAP (Simple Object Access Protocol), with REST being the most widely used.
Getting Started with the requests
Library
Installing the requests
Library
Before using the library, ensure it’s installed in your Python environment. You can install it using pip
:
pip install requests
Importing the Library
To start working with APIs, import the library:
import requests
Sending HTTP Requests
APIs communicate via HTTP, and the requests
library supports various HTTP methods, including:
GET: Retrieve data from a server.
POST: Send data to a server to create or update a resource.
PUT: Update or replace an existing resource.
DELETE: Remove a resource.
Let’s explore these methods in detail.
1. Making a GET Request
A GET request is used to retrieve data from an API endpoint.
Example: Fetching Data from a Public API
import requests
url = "https://jsonplaceholder.typicode.com/posts"
response = requests.get(url)
# Check the response status code
if response.status_code == 200:
print("Success!")
print(response.json()) # Parse JSON data
else:
print(f"Failed with status code: {response.status_code}")
Output:
[
{
"userId": 1,
"id": 1,
"title": "sunt aut facere repellat provident occaecati",
"body": "quia et suscipit\nsuscipit recusandae..."
},
...
]
Query Parameters
You can pass query parameters to an API using the params
argument.
params = {"userId": 1}
response = requests.get(url, params=params)
print(response.json())
2. Sending a POST Request
A POST request is used to send data to an API, often to create a resource.
Example: Submitting Data
url = "https://jsonplaceholder.typicode.com/posts"
data = {
"title": "New Post",
"body": "This is the content of the post",
"userId": 1
}
response = requests.post(url, json=data)
if response.status_code == 201:
print("Post created successfully!")
print(response.json())
else:
print("Failed to create post.")
Output:
{
"id": 101,
"title": "New Post",
"body": "This is the content of the post",
"userId": 1
}
3. Updating Data with PUT
A PUT request updates an existing resource.
Example: Updating a Post
url = "https://jsonplaceholder.typicode.com/posts/1"
data = {"title": "Updated Post Title"}
response = requests.put(url, json=data)
if response.status_code == 200:
print("Post updated successfully!")
print(response.json())
else:
print("Failed to update post.")
4. Deleting Data with DELETE
A DELETE request removes a resource.
Example: Deleting a Post
url = "https://jsonplaceholder.typicode.com/posts/1"
response = requests.delete(url)
if response.status_code == 200:
print("Post deleted successfully!")
else:
print("Failed to delete post.")
Handling Responses
1. Checking Status Codes
Every HTTP response has a status code:
200: Success
201: Resource created
400: Bad request
401: Unauthorized
404: Not found
500: Server error
You can check the status code using response.status_code
.
2. Parsing JSON Data
APIs often return data in JSON format, which can be parsed using response.json()
.
data = response.json()
print(data["title"])
3. Accessing Response Headers
You can inspect headers using response.headers
.
print(response.headers["Content-Type"])
Working with Authentication
Some APIs require authentication, such as API keys or tokens.
Example: Using an API Key
url = "https://api.example.com/data"
headers = {"Authorization": "Bearer YOUR_API_KEY"}
response = requests.get(url, headers=headers)
print(response.json())
Handling Errors
The requests
library raises exceptions for HTTP errors. Use try-except
to handle them gracefully.
Example: Handling Errors
try:
response = requests.get("https://api.example.com/data")
response.raise_for_status() # Raise an error for bad responses
except requests.exceptions.HTTPError as e:
print(f"HTTP error occurred: {e}")
except requests.exceptions.RequestException as e:
print(f"Error occurred: {e}")
Advanced Features
1. Timeout
To prevent your program from hanging indefinitely, set a timeout.
response = requests.get("https://api.example.com/data", timeout=5)
2. Session Objects
Use Session
for persistent settings across multiple requests.
session = requests.Session()
session.headers.update({"Authorization": "Bearer YOUR_API_KEY"})
response = session.get("https://api.example.com/data")
3. Streaming Large Responses
For large responses, process data in chunks using stream=True
.
response = requests.get("https://api.example.com/largefile", stream=True)
for chunk in response.iter_content(chunk_size=1024):
print(chunk)
Real-World Use Case: Fetching Weather Data
Example: Using OpenWeatherMap API
import requests
API_KEY = "YOUR_API_KEY"
city = "London"
url = f"http://api.openweathermap.org/data/2.5/weather?q={city}&appid={API_KEY}"
response = requests.get(url)
if response.status_code == 200:
weather_data = response.json()
print(f"Temperature: {weather_data['main']['temp']}°C")
print(f"Weather: {weather_data['weather'][0]['description']}")
else:
print("Failed to fetch weather data.")
Best Practices for Working with APIs
Read API Documentation: Understand the API’s endpoints, parameters, and authentication.
Secure API Keys: Use environment variables to store sensitive information.
Implement Error Handling: Always check for status codes and handle exceptions.
Optimize Requests: Use query parameters and limit the data fetched to reduce overhead.
Cache Responses: Cache frequently accessed data to minimize API calls.
Conclusion
Python’s requests
library simplifies the process of working with APIs, allowing you to send HTTP requests, handle responses, and manage errors effectively. By mastering the concepts and techniques discussed in this guide, you can integrate APIs seamlessly into your Python projects.
Whether you’re fetching weather data, submitting form entries, or building a data pipeline, the requests
library equips you with the tools needed to interact with APIs like a pro.
Happy coding! 🚀