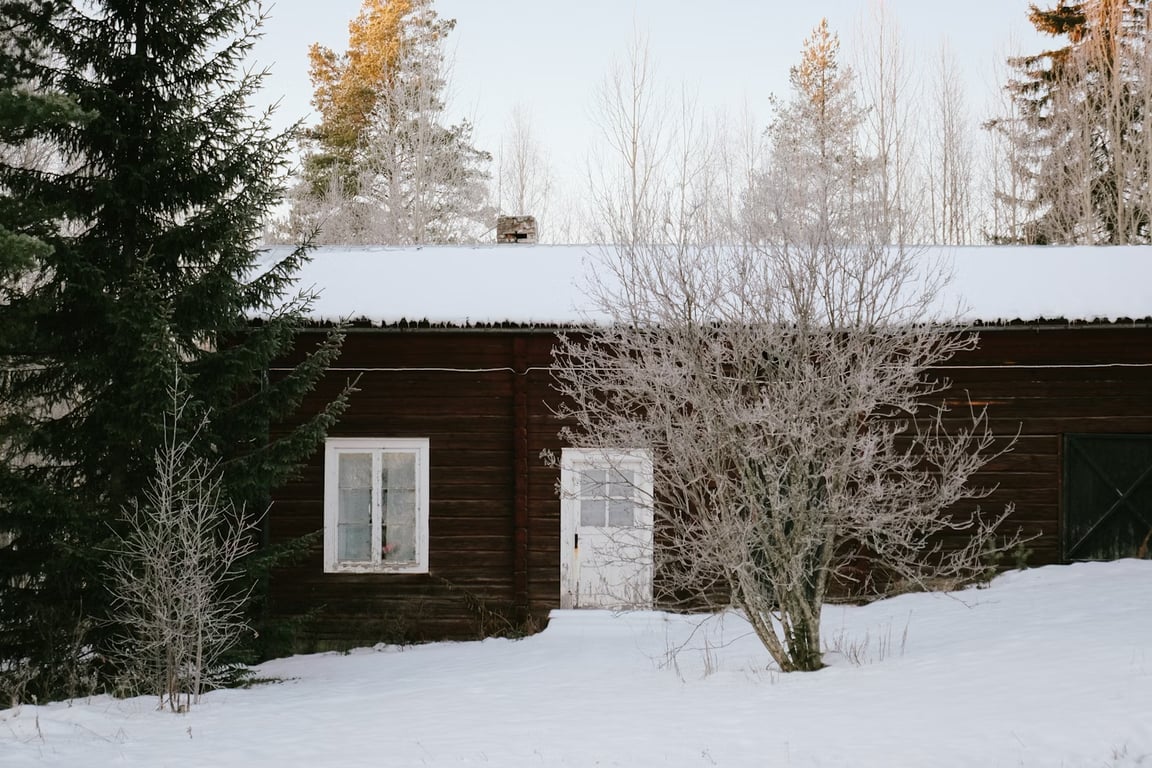
Photo by Andreas Eriksson on Unsplash
The internet is a treasure trove of data, and web scraping is a technique that allows you to extract and process this data programmatically. Python, with its vast array of libraries, makes web scraping easier and more efficient. One of the most popular libraries for web scraping in Python is Beautiful Soup, which provides tools to parse and navigate HTML or XML documents.
In this blog, we’ll dive deep into web scraping with Python and Beautiful Soup, covering everything from setup to practical examples, while also discussing best practices and ethical considerations.
What is Web Scraping?
Web scraping is the process of extracting information from websites. Instead of manually copying and pasting data, web scraping automates the task, making it faster and more efficient.
Common Use Cases of Web Scraping:
Collecting product prices for e-commerce analysis.
Gathering job postings from recruitment websites.
Extracting news headlines for sentiment analysis.
Compiling data for research projects.
Understanding the Basics of Beautiful Soup
What is Beautiful Soup?
Beautiful Soup is a Python library that makes it easy to scrape information from web pages. It parses HTML and XML documents and provides simple methods for navigating, searching, and modifying the parsed content.
Key Features of Beautiful Soup:
Easy-to-use syntax for parsing HTML.
Support for navigating and searching the document tree.
Integration with popular parsers like
html.parser
,lxml
, andhtml5lib
.
Setting Up the Environment
Before starting, ensure you have Python installed on your system. Then, install Beautiful Soup and a library like requests
to fetch web pages.
Installing Required Libraries
pip install beautifulsoup4 requests
Importing Libraries
from bs4 import BeautifulSoup
import requests
Fetching a Web Page
The first step in web scraping is fetching the HTML content of a webpage. This is typically done using the requests
library.
Example: Fetching HTML from a Website
import requests
url = "https://example.com"
response = requests.get(url)
# Check the response status
if response.status_code == 200:
print("Page fetched successfully!")
print(response.text) # HTML content of the page
else:
print("Failed to fetch the page.")
Parsing HTML with Beautiful Soup
After fetching the HTML, you can parse it with Beautiful Soup to extract specific data.
Creating a Beautiful Soup Object
from bs4 import BeautifulSoup
html_content = response.text
soup = BeautifulSoup(html_content, 'html.parser')
The soup
object now represents the parsed HTML, and you can use its methods to extract data.
Navigating the HTML Tree
Beautiful Soup provides multiple methods to navigate and search through the HTML document.
1. Accessing Elements by Tag Name
# Get the first <h1> tag
h1_tag = soup.h1
print(h1_tag.text) # Get the text content
2. Finding Elements with find()
and find_all()
# Find the first <p> tag
first_paragraph = soup.find('p')
print(first_paragraph.text)
# Find all <p> tags
all_paragraphs = soup.find_all('p')
for p in all_paragraphs:
print(p.text)
3. Using CSS Selectors with select()
# Select elements by class
elements = soup.select('.classname')
for element in elements:
print(element.text)
Extracting Data from Attributes
Often, you’ll need to extract attributes like href
from links or src
from images.
Example: Extracting Links
# Find all <a> tags and extract their href attributes
links = soup.find_all('a')
for link in links:
print(link.get('href'))
Real-World Examples
Example 1: Scraping News Headlines
url = "https://news.ycombinator.com/"
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# Extract all titles from the news page
titles = soup.find_all('a', class_='storylink')
for idx, title in enumerate(titles, start=1):
print(f"{idx}. {title.text} ({title.get('href')})")
Example 2: Scraping Product Prices
url = "https://example-ecommerce-site.com"
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# Find all product names and prices
products = soup.find_all('div', class_='product')
for product in products:
name = product.find('h2').text
price = product.find('span', class_='price').text
print(f"Product: {name}, Price: {price}")
Dealing with Dynamic Websites
Some websites use JavaScript to load content dynamically, making it difficult for Beautiful Soup to scrape the desired data. In such cases, consider using libraries like Selenium or Playwright to interact with JavaScript-rendered pages.
Example: Using Selenium
pip install selenium
from selenium import webdriver
driver = webdriver.Chrome() # Ensure you have the ChromeDriver installed
driver.get("https://example.com")
html = driver.page_source
soup = BeautifulSoup(html, 'html.parser')
Handling Common Challenges
1. Handling HTTP Errors
Use the raise_for_status()
method in requests
to detect errors.
try:
response = requests.get(url)
response.raise_for_status()
except requests.exceptions.RequestException as e:
print(f"Error: {e}")
2. Dealing with Anti-Scraping Mechanisms
Some websites employ anti-scraping measures. To bypass these:
Use headers to mimic a browser.
Add delays between requests.
Use proxies or rotating IPs.
headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36"
}
response = requests.get(url, headers=headers)
Ethical Considerations
While web scraping is powerful, it comes with ethical responsibilities:
Check the Terms of Service: Ensure the website permits web scraping.
Avoid Overloading Servers: Limit the frequency of requests to avoid burdening the server.
Use Data Responsibly: Respect privacy and intellectual property rights.
Best Practices for Web Scraping
Start Small: Scrape a small amount of data first to test your code.
Use Logging: Keep track of your scraping activities for debugging and monitoring.
Store Data Efficiently: Save data in structured formats like CSV, JSON, or databases.
Avoid Hardcoding: Use configuration files or environment variables for URLs and credentials.
Saving Scraped Data
After extracting data, you’ll often need to save it for further analysis.
Example: Saving Data to CSV
import csv
data = [{"name": "Product A", "price": "$10"}, {"name": "Product B", "price": "$20"}]
with open("products.csv", "w", newline="") as file:
writer = csv.DictWriter(file, fieldnames=["name", "price"])
writer.writeheader()
writer.writerows(data)
Conclusion
Web scraping with Python and Beautiful Soup is a valuable skill for extracting and analyzing data from websites. With the tools and techniques discussed in this guide, you can build your own web scraping projects, from simple data extraction to complex automation tasks.
Remember to always scrape responsibly and adhere to ethical guidelines. Happy scraping!
Happy coding!