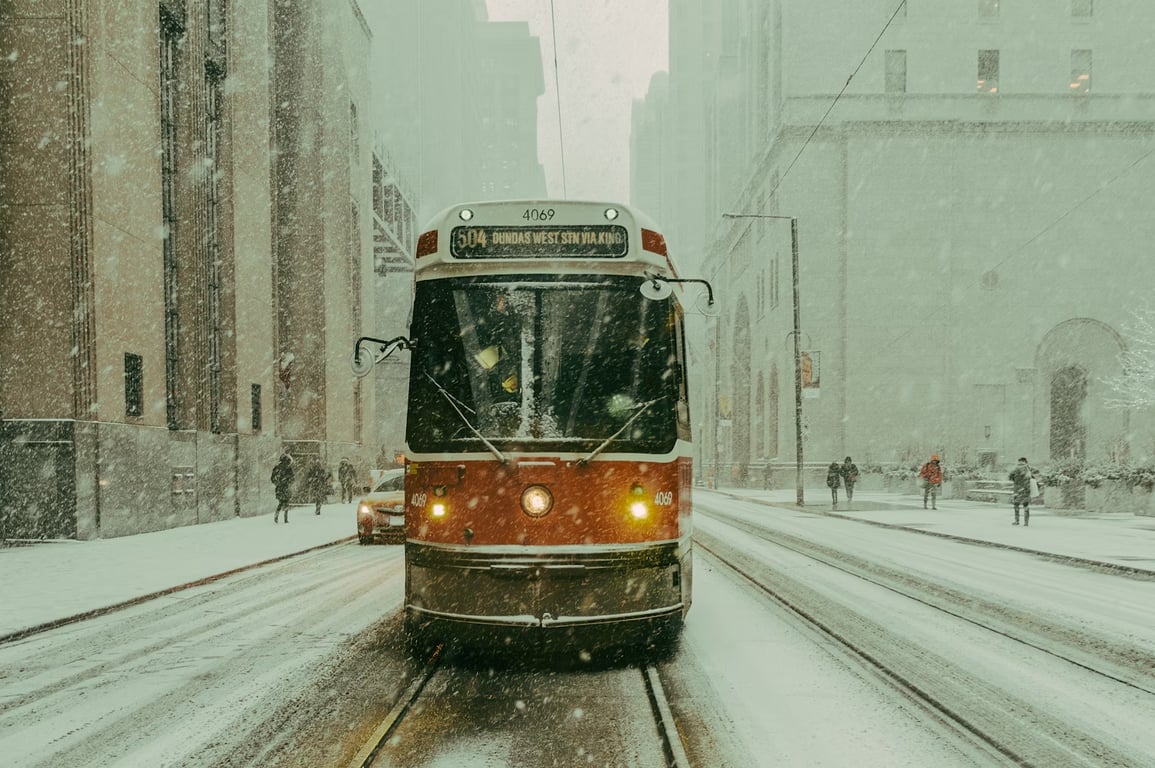
Photo by Kyle Hinkson on Unsplash
Table of Contents
Introduction
What is HTMX?
Why Use HTMX with Django?
Setting Up HTMX in a Django Project
Handling HTMX Requests in Django Views
Example: Creating a Live Search Feature with HTMX
Example: Implementing Infinite Scrolling with HTMX
Handling Form Submissions Dynamically
Enhancing User Experience with HTMX
Conclusion
1. Introduction
Django is a powerful web framework for building dynamic applications. Traditionally, JavaScript frameworks like React or Vue are used to enhance interactivity. However, HTMX provides an alternative approach, enabling dynamic web applications without writing JavaScript.
With HTMX, you can make AJAX requests, update parts of a page, and handle user interactions—all while keeping your Django templates clean and simple.
2. What is HTMX?
HTMX is a lightweight JavaScript library that allows you to send AJAX requests and update elements on a webpage without writing JavaScript manually. It extends HTML with additional attributes like:
hx-get
→ Send a GET request when an element is clicked.hx-post
→ Send a POST request when a form is submitted.hx-target
→ Specify which part of the page should be updated.hx-swap
→ Define how the response should be inserted (e.g., replace, before, after).
Example: Fetching data without JavaScript
<button hx-get="/fetch-data/" hx-target="#result">Fetch Data</button>
<div id="result"></div>
3. Why Use HTMX with Django?
Using HTMX with Django offers several benefits:
✅ No JavaScript Required – Keep your frontend logic inside Django views and templates.
✅ Improved Performance – Fetch and update only necessary parts of a page.
✅ Better SEO & Accessibility – No reliance on heavy JavaScript frameworks.
✅ Simple & Maintainable – Works with Django’s existing template engine.
4. Setting Up HTMX in a Django Project
HTMX is a lightweight library, and you can include it in your Django project by adding it via CDN or installing it via npm.
Option 1: Include HTMX via CDN
Add this line to your Django template (e.g., base.html
):
<script src="https://unpkg.com/htmx.org@1.9.6"></script>
Option 2: Install HTMX via npm
npm install htmx.org
Then include it in your static files.
5. Handling HTMX Requests in Django Views
Django can handle HTMX requests just like regular HTTP requests. HTMX sends an HX-Request header, allowing you to detect and return partial HTML responses.
Example: HTMX Handling in Django Views
from django.shortcuts import render
from django.http import JsonResponse
def fetch_data(request):
if request.headers.get('HX-Request'):
return render(request, 'partials/data_snippet.html', {'message': 'Hello from Django!'})
return render(request, 'main_page.html')
6. Example: Creating a Live Search Feature with HTMX
Step 1: Create a Django View for Searching
from django.shortcuts import render
from myapp.models import Product
def live_search(request):
query = request.GET.get('q', '')
results = Product.objects.filter(name__icontains=query)
return render(request, 'partials/search_results.html', {'results': results})
Step 2: Create an HTMX-Enabled Search Form
<input type="text" hx-get="/search/" hx-trigger="keyup changed delay:300ms" hx-target="#search-results">
<div id="search-results"></div>
Step 3: Create a Partial Template (partials/search_results.html
)
{% for product in results %}
<p>{{ product.name }}</p>
{% empty %}
<p>No results found.</p>
{% endfor %}
Now, as the user types in the search bar, HTMX dynamically fetches matching results without reloading the page. 🚀
7. Example: Implementing Infinite Scrolling with HTMX
Step 1: Create a Django View for Paginated Data
from django.core.paginator import Paginator
from django.shortcuts import render
from myapp.models import Article
def load_more_articles(request):
page = int(request.GET.get('page', 1))
articles = Article.objects.all()
paginator = Paginator(articles, 5)
articles_page = paginator.get_page(page)
return render(request, 'partials/articles.html', {'articles': articles_page})
Step 2: Create an HTMX-Enabled Load More Button
<div id="article-list">
{% include 'partials/articles.html' %}
</div>
<button hx-get="/load-more/?page=2" hx-target="#article-list" hx-swap="beforeend">
Load More
</button>
HTMX will fetch the next batch of articles dynamically when the user clicks Load More.
8. Handling Form Submissions Dynamically
Step 1: Django View for Handling Form Submission
from django.shortcuts import render
from myapp.forms import ContactForm
def submit_form(request):
form = ContactForm(request.POST or None)
if form.is_valid():
form.save()
return render(request, 'partials/success_message.html')
return render(request, 'partials/contact_form.html', {'form': form})
Step 2: HTMX-Enabled Form
<form hx-post="/submit-form/" hx-target="#form-response">
{% csrf_token %}
{{ form.as_p }}
<button type="submit">Submit</button>
</form>
<div id="form-response"></div>
Step 3: Partial Templates for Responses
✅ partials/contact_form.html
(for re-rendering the form if there are errors)
✅ partials/success_message.html
(for showing a success message)
HTMX updates only the target #form-response, making the form submission dynamic without reloading the page.
9. Enhancing User Experience with HTMX
HTMX provides additional features to improve UX:
Triggering Requests on Hover
<div hx-get="/hover-content/" hx-trigger="mouseover" hx-target="#hover-result"></div>
<div id="hover-result"></div>
Disabling Buttons After Submission
<button hx-post="/submit/" hx-disabled-elt="true">Submit</button>
Adding Loading Indicators
<button hx-get="/fetch-data/" hx-target="#data" hx-indicator="#loading">Fetch Data</button>
<div id="loading" style="display: none;">Loading...</div>
<div id="data"></div>
HTMX simplifies frontend interactivity while keeping your Django code clean.
10. Conclusion
HTMX allows developers to build dynamic Django applications without JavaScript, leveraging Django's existing template system to create fast, maintainable, and interactive web applications.
Key Takeaways:
✅ HTMX makes AJAX-like requests without writing JavaScript.
✅ Django can return partial HTML responses for seamless updates.
✅ Live search, infinite scrolling, and dynamic forms become trivial.
✅ HTMX improves performance and user experience with minimal effort.
By integrating Django with HTMX, you can create modern, interactive applications without relying on heavy JavaScript frameworks! 🚀