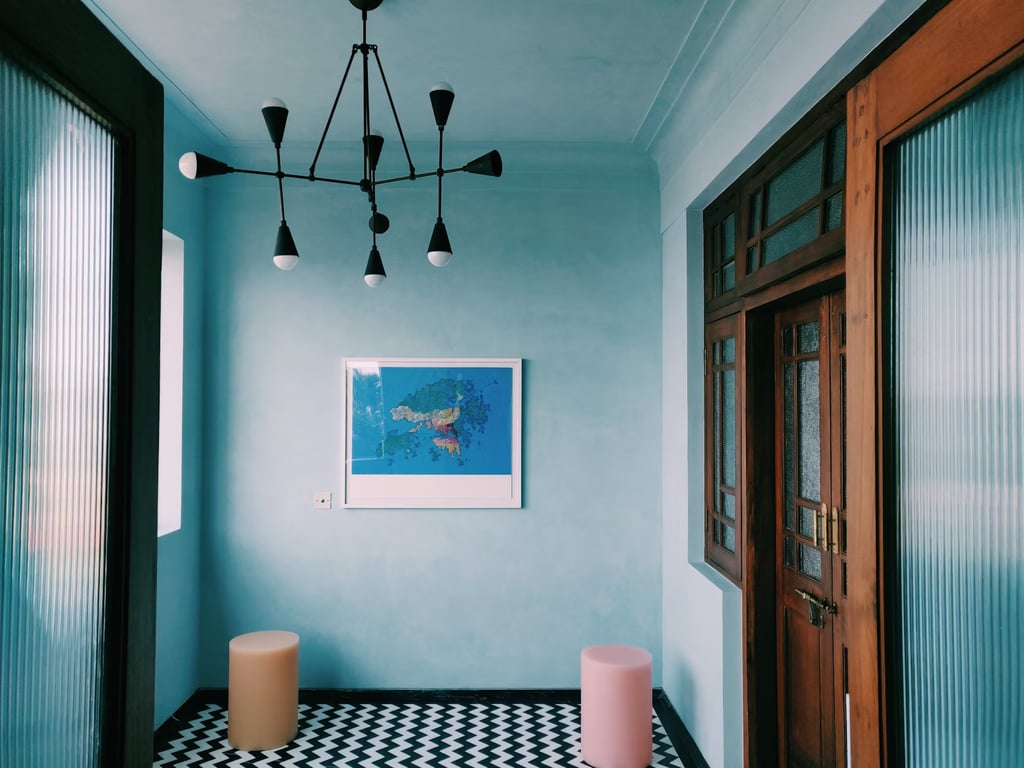
Photo by Sagar Sawant on Unsplash
Table of Contents
Introduction
Understanding Django ORM
Common Performance Issues in Django ORM
Best Practices for Optimizing Django ORM Queries
4.1 Use
select_related
andprefetch_related
4.2 Avoid N+1 Query Problem
4.3 Use Indexing for Faster Lookups
4.4 Optimize Queries with QuerySet Methods
4.5 Use
only()
anddefer()
to Limit Data Loading4.6 Reduce Query Count with
annotate()
andaggregate()
4.7 Optimize Pagination with
limit()
andoffset()
4.8 Cache Query Results
Profiling and Debugging Django Queries
Conclusion
1. Introduction
Django’s ORM (Object-Relational Mapper) provides a powerful abstraction layer for interacting with databases. While it simplifies database queries, improper usage can lead to significant performance bottlenecks. In this guide, we will explore techniques to optimize Django ORM queries, making your application lightning-fast.
2. Understanding Django ORM
Django ORM allows developers to interact with the database using Python instead of writing raw SQL queries. This abstraction helps improve maintainability, but it can also introduce inefficiencies if queries are not optimized properly.
A basic Django ORM query looks like this:
from myapp.models import Product
products = Product.objects.all()
While this seems simple, running such queries inefficiently can slow down your application, especially when dealing with large datasets.
3. Common Performance Issues in Django ORM
Before optimizing, let’s understand common performance problems:
N+1 query problem: Fetching related objects in a loop instead of a single query.
Unnecessary data loading: Fetching all fields when only a few are needed.
Lack of indexing: Queries running without proper indexes on columns.
Excessive query counts: Running multiple queries when a single optimized query can be used.
Inefficient pagination: Fetching thousands of records when only a few are displayed.
Now, let's explore best practices for optimizing Django ORM queries.
4. Best Practices for Optimizing Django ORM Queries
4.1 Use select_related
and prefetch_related
Django provides select_related
and prefetch_related
to optimize queries involving related objects.
select_related
: Uses SQL joins to retrieve related data in a single query (recommended for ForeignKey relationships).prefetch_related
: Fetches related objects separately and then links them in Python (useful for ManyToMany relationships).
# This will trigger multiple queries for each author
books = Book.objects.all()
for book in books:
print(book.author.name)
Optimized Query with select_related
books = Book.objects.select_related('author').all()
for book in books:
print(book.author.name) # Fetches data in a single query
Optimized Query with prefetch_related
books = Book.objects.prefetch_related('categories').all()
for book in books:
print(book.categories.all()) # Optimized ManyToMany retrieval
4.2 Avoid N+1 Query Problem
The N+1 query problem occurs when a query is executed multiple times unnecessarily. Using select_related
and prefetch_related
(as shown above) eliminates this problem.
4.3 Use Indexing for Faster Lookups
Indexes improve database performance by speeding up lookups. Add indexes to frequently queried fields.
Example: Adding an Index to a Fieldfrom django.db import models
class User(models.Model):
email = models.EmailField(unique=True, db_index=True) # Adding index
For existing models, run:
python manage.py makemigrations
python manage.py migrate
4.4 Optimize Queries with QuerySet Methods
Django provides methods like exists()
, count()
, values()
, and values_list()
to optimize queries.
exists()
Instead of CountingBad Practice:
if User.objects.filter(email="test@example.com").count() > 0:
print("User exists")
Optimized Query:
if User.objects.filter(email="test@example.com").exists():
print("User exists") # Executes a more efficient SQL query
Example: Using values_list()
Instead of Fetching Entire Objects
# Inefficient: Fetches full objects
usernames = [user.username for user in User.objects.all()]
# Optimized: Fetches only the required field
usernames = list(User.objects.values_list('username', flat=True))
4.5 Use only()
and defer()
to Limit Data Loading
When querying large models, avoid loading unnecessary fields.
Example: Fetching Only Required Fields# Loads only name and email fields
users = User.objects.only("name", "email")
Example: Deferring Unnecessary Fields
users = User.objects.defer("profile_picture", "bio") # Skips loading large fields
4.6 Reduce Query Count with annotate()
and aggregate()
Instead of running multiple queries, use annotate()
and aggregate()
to perform calculations at the database level.
from django.db.models import Count
authors = Author.objects.annotate(book_count=Count('books'))
for author in authors:
print(author.name, author.book_count) # Fetches book count efficiently
Example: Aggregating Data
from django.db.models import Avg
average_price = Product.objects.aggregate(Avg('price'))
print(average_price) # {'price__avg': 200.50}
4.7 Optimize Pagination with limit()
and offset()
When paginating data, avoid loading all records at once.
Example: Usinglimit()
and offset()
products = Product.objects.all()[10:20] # Fetches only 10 records
Using Django’s built-in Paginator
:
from django.core.paginator import Paginator
products = Product.objects.all()
paginator = Paginator(products, 10) # 10 items per page
page1 = paginator.page(1) # Get first page
4.8 Cache Query Results
Use Django’s caching framework to store frequently accessed queries.
Example: Caching Query Resultsfrom django.core.cache import cache
products = cache.get("products")
if not products:
products = Product.objects.all()
cache.set("products", products, timeout=600) # Cache for 10 minutes
5. Profiling and Debugging Django Queries
Use Django Debug Toolbar to analyze queries in development.
Install Django Debug Toolbarpip install django-debug-toolbar
Add it to INSTALLED_APPS
and middleware in settings.py
:
INSTALLED_APPS += ["debug_toolbar"]
MIDDLEWARE += ["debug_toolbar.middleware.DebugToolbarMiddleware"]
INTERNAL_IPS = ["127.0.0.1"]
Run the server, open Django Admin and check the queries in the debug panel.
6. Conclusion
Optimizing Django ORM queries is crucial for improving application performance. By following these best practices—such as using select_related()
, prefetch_related()
, indexing, and caching—you can significantly speed up database queries and enhance the user experience.
By continuously profiling and optimizing queries, you ensure your Django application remains scalable and efficient. 🚀
Happy coding!