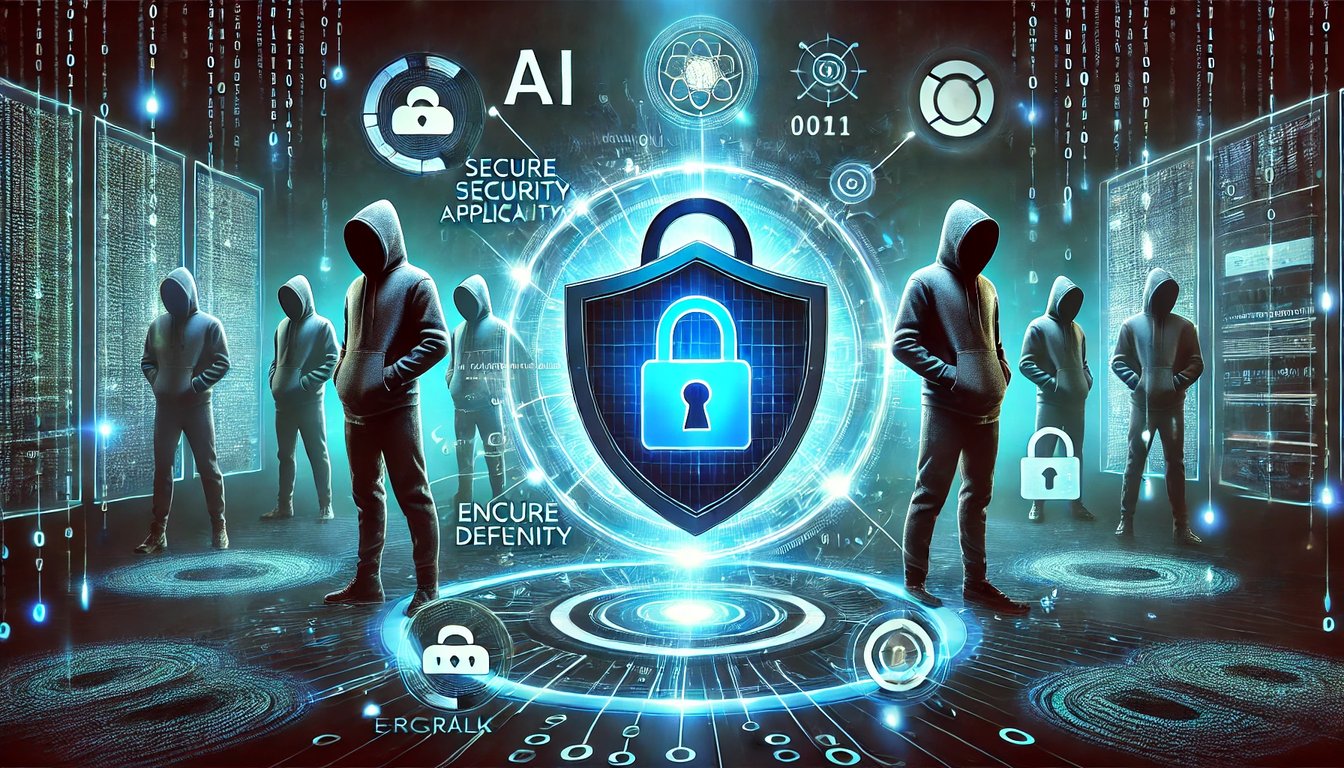
Cyber threats are evolving rapidly, and securing Django applications is more critical than ever in 2025. Hackers use sophisticated techniques to exploit web applications, making it essential to follow best security practices.
This guide will cover Django security best practices to protect your web app from SQL injection, XSS, CSRF, authentication flaws, data leaks, and more.
Table of Contents
Introduction
Why Security Matters in 2025
Keeping Django and Dependencies Updated
Preventing SQL Injection
Protecting Against Cross-Site Scripting (XSS)
Securing Against Cross-Site Request Forgery (CSRF)
Strengthening User Authentication
Securing Sensitive Data and Environment Variables
Implementing HTTPS and Secure Cookies
Setting Up Django Security Middleware
Using Content Security Policy (CSP)
Enabling Logging and Monitoring for Security Threats
Using Web Application Firewalls (WAF)
Deploying Django Apps Securely
Conclusion
1. Introduction
With growing cyber threats, Django developers must proactively secure their applications. A single vulnerability can expose sensitive data, allow account takeovers, or compromise an entire system.
This guide outlines practical security measures every Django developer should implement to protect web applications from modern threats.
2. Why Security Matters in 2025
π¨ Key Cyber Threats in 2025
AI-powered hacking tools can automate SQL injection and XSS attacks.
Ransomware attacks targeting web applications are on the rise.
Zero-day vulnerabilities in open-source packages are becoming more frequent.
Implementing robust security measures is necessary to safeguard user data and ensure compliance with regulations like GDPR, CCPA, and OWASP guidelines.
3. Keeping Django and Dependencies Updated
β Why it Matters?
Outdated Django versions and third-party packages contain vulnerabilities that hackers can exploit.
π Security Best Practice:
Always use the latest Django LTS version (Long-Term Support).
Regularly update dependencies using:
pip list --outdated
pip install --upgrade package_name
Use Dependabot or pip-audit to check for vulnerabilities:
pip install pip-audit
pip-audit
4. Preventing SQL Injection
π¨ Threat:Attackers manipulate database queries to steal, modify, or delete data.
π How to Prevent SQL Injection?
β Always use Django ORM instead of raw SQL queries:
# β
Safe query using Django ORM
user = User.objects.get(username="john_doe")
# β Unsafe raw SQL query (prone to SQL injection)
cursor.execute(f"SELECT * FROM users WHERE username = '{user_input}'")
5. Protecting Against Cross-Site Scripting (XSS)
π¨ Threat:Hackers inject malicious JavaScript into web pages to steal user data or hijack sessions.
π How to Prevent XSS?
β Django auto-escapes template output:
<!-- β
Safe: Django escapes the variable automatically -->
<p>{{ user_input }}</p>
β
Avoid using safe
filter unless necessary:
<!-- β Dangerous: Allows XSS attacks -->
<p>{{ user_input|safe }}</p>
β
Use Django's bleach
package to sanitize HTML input:
from bleach import clean
safe_input = clean(user_input)
6. Securing Against Cross-Site Request Forgery (CSRF)
π¨ Threat:Hackers trick users into performing unauthorized actions on your site.
π How to Prevent CSRF?
β Django automatically protects against CSRF in forms:
<form method="POST">
{% csrf_token %}
<input type="text" name="comment">
<button type="submit">Submit</button>
</form>
β Ensure CSRF protection is enabled in settings.py:
MIDDLEWARE = [
"django.middleware.csrf.CsrfViewMiddleware", # Must be enabled
]
β Use CSRF exemptions cautiously when working with APIs:
from django.views.decorators.csrf import csrf_exempt
@csrf_exempt
def my_view(request):
return HttpResponse("CSRF not required here")
7. Strengthening User Authentication
π¨ Threat:Weak authentication allows account takeovers and brute-force attacks.
π How to Secure User Authentication?
β Use Djangoβs built-in authentication system:
from django.contrib.auth.models import User
user = User.objects.create_user(username="john_doe", password="secure_password123")
β Enforce strong password policies in settings.py:
AUTH_PASSWORD_VALIDATORS = [
{"NAME": "django.contrib.auth.password_validation.MinimumLengthValidator"},
{"NAME": "django.contrib.auth.password_validation.CommonPasswordValidator"},
]
β Enable Two-Factor Authentication (2FA) using Django-AllAuth.
8. Securing Sensitive Data and Environment Variables
π¨ Threat:Exposing database credentials or API keys can lead to data breaches.
π Best Practices:
β
Store sensitive credentials in .env
files, not in settings.py
:
# .env file
SECRET_KEY="super-secret-key"
DATABASE_PASSWORD="secure-password"
β Use Django-environ to load environment variables:
import environ
env = environ.Env()
environ.Env.read_env()
SECRET_KEY = env("SECRET_KEY")
DATABASE_PASSWORD = env("DATABASE_PASSWORD")
9. Implementing HTTPS and Secure Cookies
π Enforce HTTPS in settings.py:
SECURE_SSL_REDIRECT = True
SESSION_COOKIE_SECURE = True
CSRF_COOKIE_SECURE = True
β Use HSTS (Strict-Transport-Security) to prevent protocol downgrade attacks:
SECURE_HSTS_SECONDS = 31536000 # One year
SECURE_HSTS_INCLUDE_SUBDOMAINS = True
10. Setting Up Django Security Middleware
Ensure security middleware is enabled:
MIDDLEWARE = [
"django.middleware.security.SecurityMiddleware",
"django.middleware.common.CommonMiddleware",
"django.middleware.csrf.CsrfViewMiddleware",
]
11. Using Content Security Policy (CSP)
π Prevent malicious scripts using CSP headers:
CSP_DEFAULT_SRC = ("'self'",)
CSP_SCRIPT_SRC = ("'self'", "trusted-cdn.com")
12. Enabling Logging and Monitoring for Security Threats
Use Djangoβs logging system to detect suspicious activity:
LOGGING = {
"version": 1,
"handlers": {
"file": {
"level": "WARNING",
"class": "logging.FileHandler",
"filename": "security.log",
},
},
"loggers": {
"django.security": {
"handlers": ["file"],
"level": "WARNING",
"propagate": True,
},
},
}
Conclusion
β Keeping Django updated prevents security exploits.
β Protect against SQL injection, XSS, and CSRF attacks.
β Secure user authentication and environment variables.
β Enforce HTTPS, CSP, and security middleware.
By implementing these security practices, you can protect your Django app from hackers in 2025 and beyond. π