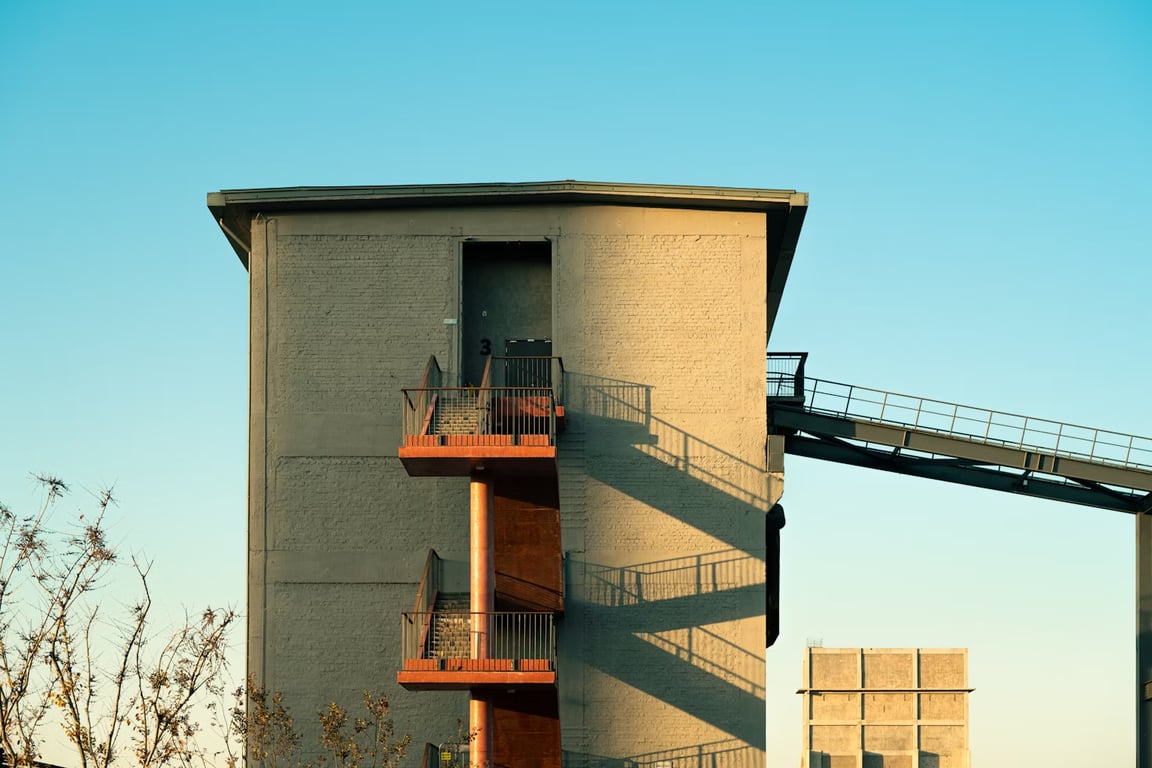
Python is one of the most widely used programming languages due to its simplicity, versatility, and extensive community support. However, one aspect of Python that often confuses beginners and experienced developers alike is its Global Interpreter Lock (GIL). Understanding the GIL and its impact on multithreading is crucial, especially if you’re working on performance-critical applications.
In this blog, we’ll take an in-depth look at Python’s GIL, explain what it is, how it works, and explore its effect on multithreading. We’ll also discuss alternatives and strategies to bypass or mitigate the limitations introduced by the GIL, and understand how Python's concurrency model fits into modern applications.
What is the Global Interpreter Lock (GIL)?
The Global Interpreter Lock (GIL) is a mechanism used in Python’s CPython implementation to ensure that only one thread can execute Python bytecode at a time. This means that even if a program has multiple threads, only one thread can run Python code at a time, while the other threads are blocked and waiting for the GIL to be released.
Why Does Python Have a GIL?
The GIL was introduced to make CPython (the most common implementation of Python) easier to implement. CPython is not inherently thread-safe, and without the GIL, managing memory and access to objects in multi-threaded environments could result in race conditions and data corruption. The GIL simplifies the process of memory management and ensures that only one thread accesses the interpreter at a time.
However, this convenience comes at a cost—especially when trying to take advantage of multi-core processors in multi-threaded programs.
How the GIL Affects Multithreading in Python
1. Single-threaded Execution with Python Threads
Even though you can create multiple threads in Python using the threading
module, the presence of the GIL prevents these threads from executing Python bytecode simultaneously. This means that in CPU-bound tasks, Python threads do not offer performance improvements. Instead, you’ll find that your program runs with a single thread, even if you’ve created multiple threads.
For instance, if you have a program that performs computationally expensive tasks (e.g., calculations, data processing), the threads will execute sequentially, each waiting for the GIL to be released before it can run.
Example: GIL and CPU-bound TaskLet’s consider an example where we try to perform a CPU-bound task (e.g., summing large numbers) using threads:
import threading
import time
# CPU-bound function: summing numbers
def sum_numbers(start, end):
result = 0
for i in range(start, end):
result += i
print(f"Result: {result}")
def thread_task():
start_time = time.time()
threads = []
# Create multiple threads
for _ in range(5):
t = threading.Thread(target=sum_numbers, args=(0, 10**7))
threads.append(t)
t.start()
# Join all threads
for t in threads:
t.join()
end_time = time.time()
print(f"Multithreading Execution Time: {end_time - start_time:.4f} seconds")
if __name__ == "__main__":
thread_task()
In the example above, you might not see a significant improvement in execution time, despite running multiple threads, because the GIL only allows one thread to execute Python code at any given moment.
2. I/O-bound Tasks and Python Multithreading
Unlike CPU-bound tasks, Python’s multithreading shines in scenarios that are I/O-bound. These include tasks like file I/O, network I/O, or database queries, where threads spend a significant amount of time waiting for I/O operations to complete. In this case, while one thread is waiting for an I/O operation to complete, the GIL can be released, allowing other threads to execute.
Example: GIL and I/O-bound TaskLet’s see how the GIL affects multithreading when working with I/O-bound tasks like downloading multiple web pages:
import threading
import requests
import time
# Function to download a webpage
def download_page(url):
response = requests.get(url)
print(f"Downloaded {url}, Status Code: {response.status_code}")
def thread_task():
urls = [
"https://www.example.com",
"https://www.google.com",
"https://www.python.org",
"https://www.github.com"
]
start_time = time.time()
threads = []
# Create multiple threads to download pages
for url in urls:
t = threading.Thread(target=download_page, args=(url,))
threads.append(t)
t.start()
# Join all threads
for t in threads:
t.join()
end_time = time.time()
print(f"Multithreading Execution Time: {end_time - start_time:.4f} seconds")
if __name__ == "__main__":
thread_task()
In this case, multithreading can speed up the program because while one thread is waiting for a webpage to download, other threads can run. The GIL doesn’t cause much of a performance bottleneck for I/O-bound tasks.
Alternatives to Python’s GIL
1. Using Multiprocessing for CPU-bound Tasks
For CPU-bound tasks, Python’s multiprocessing
module is a better alternative. Instead of threads, it uses processes, which each have their own memory space and interpreter, bypassing the GIL limitation. With multiple processes, Python can take full advantage of multi-core processors, which is particularly useful for parallelizing CPU-intensive tasks.
import multiprocessing
import time
# CPU-bound function: summing numbers
def sum_numbers(start, end):
result = 0
for i in range(start, end):
result += i
print(f"Result: {result}")
def process_task():
start_time = time.time()
processes = []
# Create multiple processes
for _ in range(5):
p = multiprocessing.Process(target=sum_numbers, args=(0, 10**7))
processes.append(p)
p.start()
# Join all processes
for p in processes:
p.join()
end_time = time.time()
print(f"Multiprocessing Execution Time: {end_time - start_time:.4f} seconds")
if __name__ == "__main__":
process_task()
Using multiprocessing
, each process runs independently and can execute on separate CPU cores. This enables full utilization of multi-core systems for CPU-bound tasks, unlike multithreading, which is limited by the GIL.
2. Using Jython or IronPython
If your application requires true multithreading and you need to bypass the GIL, you can consider using alternative Python implementations like Jython or IronPython. These implementations do not have the GIL and are better suited for multi-threading on multi-core systems.
Jython is a Java-based implementation of Python that runs on the Java Virtual Machine (JVM).
IronPython is an implementation of Python for the .NET framework.
Both implementations can execute Python code in parallel on multiple threads without the restrictions of the GIL.
3. Using Cython
Cython is another approach where you can compile Python code into C extensions. By using Cython, you can release the GIL in specific parts of your code to allow true parallel execution. This is especially useful for performance-critical sections of code, such as numerical computations or tight loops.
Conclusion: The GIL and Its Impact on Python Multithreading
Python's GIL is a necessary evil for CPython to manage memory and objects in a multi-threaded environment. While it can be a significant limitation for CPU-bound tasks, it doesn’t affect I/O-bound tasks as much, and Python's multithreading model works fine for tasks that involve waiting on external resources.
If your application needs to make use of multiple CPU cores, the multiprocessing
module is your best bet, as it bypasses the GIL by using processes instead of threads. If you’re dealing with I/O-bound tasks, Python’s threading
module can still provide speed improvements by allowing other threads to execute while waiting for I/O operations.
Ultimately, understanding the GIL and its implications will help you make the best decisions about concurrency in Python, ensuring that your applications are both efficient and scalable.
Happy coding!